Introduction
Let us understand what a datatype is, before we start learning about Enumeration in C. Datatype in any programming language is the collection or group of data with the values which has fixed characteristics. There are three different datatypes generally in ANSI C. They are primary, derived and user defined datatypes. Primary or built-in datatype includes int,char,double,float and void. Secondary or derived datatype includes array,references, pointers whereas user defined datatype can include Structure, Enumeration and Union.
Let us learn about Enum as a part of this blog post.
Enumeration in C
Enumeration is a user defined datatype in C. It is used to assign names to integral constant and to define an enumeration, keyword enum is used.
Syntax of enum is,
enum {constant1, constant2,………….,constantN};
Different types of declaration is possible in enum.
Type 1:
enum colors {
red,
blue,
orange };
Type 2:
enum bool {false,true};
enum bool var;
(or)
enum bool {
false, true
}var;
Now that we have seen the different types of declarations of variables using enum, we will go through various types of initialization of enum elements.
Initializations
Example 1
User can assign values to enum names explicitly while declaring them. If not assigned, compiler will assign values to the enum constants from 0.
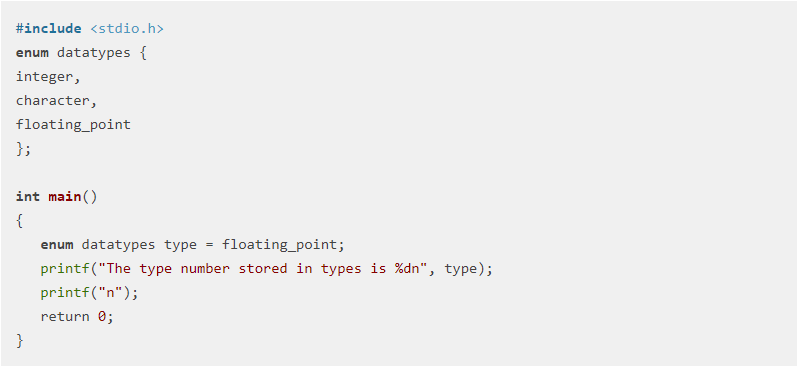
Output

In the above given example, values to the enum names are not explicitly assigned. Hence when we try to print floating_point in line 11, the value printed is 2. Thus, compiler has assigned values to the constants as follows, Integer = 0, character = 1, floating_point = 2.
Example 2
User can assign any value to any names in any order. The name for which value is not assigned will take the value of its previous name added by 1.
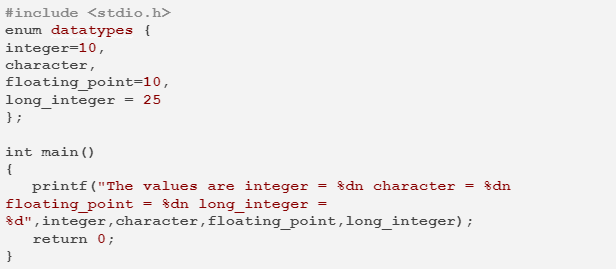
Output

In the above given example, enum constant character in line 4 takes value of integer in line 3 added by 1. Hence when we print character we get the value as 11. Also if we observe the values assigned to integer and floating point in line 3 and line 5, it is 10. Hence we can have same value assigned to two different names.
Example 3
Any enum constant should be defined/declared once in a file, it means the constant should be unique in their scope. User cannot define same name in multiple enums under a same file.
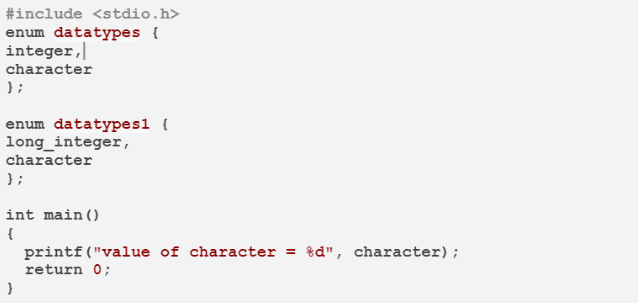
Output

n the above given example, character declared in two different enum under same file. Hence compiler flags an error saying character has been defined twice.
Enum vs Macro
Programmer can improve the readability of code using Enums. Here is an example which explains it completely. Consider the below macro declaration where 3 different macros are declared with values 0,1 and 2.
Example
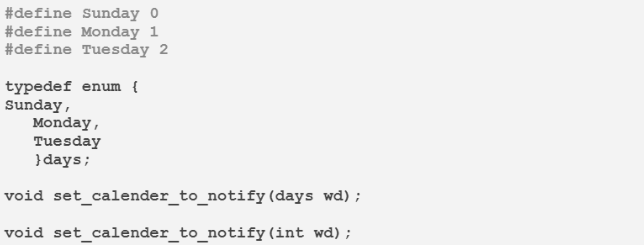
It is easier to read a code like one written in line 11 rather than the code in line 13. It is because in a big program, where there are more number of lines of code and if we use enum we will get to know what can be passed to argument parameters. Also, we don’t have to explicitly assign any values to enum constants during declaration like Macros.
Though readability is achieved using enums, we cannot define some values using enums. Using macros, we can define PI as 3.14. If the same is done using enums by providing values explicitly, compiler throws an error.
Output

Scope rule
Enum follows scope rule unlike macros. Hence if any change is made to enum constants within a scope, it will not be reflected outside the scope. Here in the below given example, compiler assigns integer 1 to enumerator true_val in line 5.
When the value is explicitly assigned to true_val within a scope in line 13, we could observe that the value is reflected in the print statement which is present within the scope (line 14), not in line 16.
This is helpful when we write a project which contains more number of codes, where if we need to change value of an enumerator, we can have the luxury of changing it, since it won’t be reflected anywhere else other than that particular scope.
Example
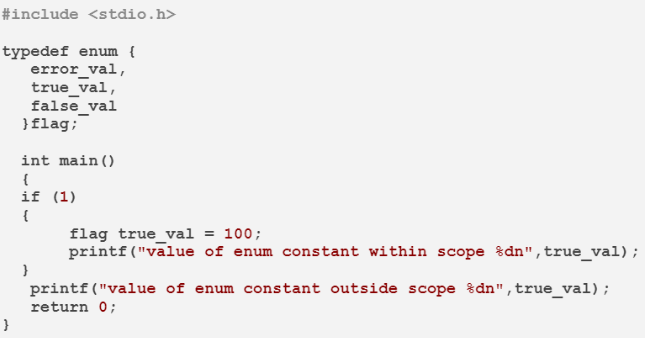
Output

Enum and Switch Statement
Enum constants can be used in switch statements by providing the symbolic names to case identifiers.
Example
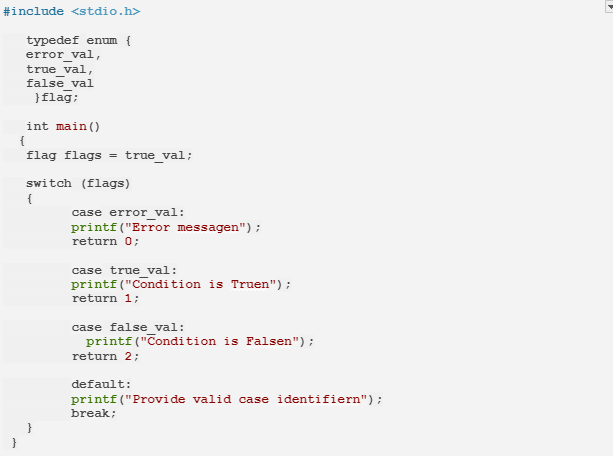
Output

In the above given example, we could observe that the case identifiers in line 15,19 and 23 are enum constants. When any one of the cases becomes true, the respective print statement executed. Since we have passed true_val to flags in line 11, the print statement under case true_val (line 20) got executed.
Conclusion
Enum being the user defined data type which consists of integral constants, is very helpful in increasing the code readability, thus decreasing the time taken for understanding the code.
Though enum increases readability, we can’t use enums for bitwise operations where an unsigned type is required. It is because enums are signed and they work well when user doesn’t have to care about the actual value of it.