QT Programming | Sample Programs
QT Programming – Sample Programs
Sample programs is the first step you take to transition from theory to practical. These sample programs typically demonstrated in a classroom will ensure you are able to immediately see it running in front of your eyes. Added to that our mentors will provide you some QT programming examples with exercises where you will be modifying the code in the class itself. By doing this fill-in-the-blank approach, will take out your fear of coding.
Chapter 1 : Fundamentals of Qt Modules
Brief:
A simple Project to print “Hello World” using Qt.
HelloWorld.pro:
QT += widgets
TEMPLATE = app
SOURCES = main.cpp
Main File:
/*---------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 Mar 2016 16:00:04 IST
* File : qt_t001_ch1_hello_world
* Title : First Qt project
* Description : A simple "Hello world" project
*--------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QPushButton button("Hello world");
button.show();
return app.exec();
}
QT programming Example output:


Chapter 2 : Core Classes
Brief:
/*-------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 Mar 2021 16:00:04 IST
* File : simple_window.h
* Title : Create a class named simpleWindow and inherit QWidget to create the window
* Description : A header file to define the class named SimpleWindow
*------------------------------------------------------------------------------------------------------------*/
#ifndef SIMPLEWINDOW_H
#define SIMPLEWINDOW_H
#include
class SimpleWindow : public QWidget
{
Q_OBJECT
public:
explicit SimpleWindow(QWidget *parent = 0);
};
#endif // SIMPLEWINDOW_H
Main File:
/*-------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 Mar 2021 16:00:04 IST
* File : main.cpp
* Title : Project to display simple layout
* Description : A simple layout to display text editor with 2 buttons
*-----------------------------------------------------------------------------------------------------------*/
#include "simplewindow.h"
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
SimpleWindow window;
window.show();
return app.exec();
}
MOC Simple Window:
/*------------------------------------------------------------------------------------------------------------
** Meta object code from reading C++ file 'simplewindow.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
-------------------------------------------------------------------------------------------------------------*/
#include "simplewindow.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'simplewindow.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_SimpleWindow_t {
QByteArrayData data[1];
char stringdata0[13];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_SimpleWindow_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_SimpleWindow_t qt_meta_stringdata_SimpleWindow = {
{
QT_MOC_LITERAL(0, 0, 12) // "SimpleWindow"
},
"SimpleWindow"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_SimpleWindow[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
0, 0, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
0 // eod
};
void SimpleWindow::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
Q_UNUSED(_o);
Q_UNUSED(_id);
Q_UNUSED(_c);
Q_UNUSED(_a);
}
QT_INIT_METAOBJECT const QMetaObject SimpleWindow::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_SimpleWindow.data,
qt_meta_data_SimpleWindow,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *SimpleWindow::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *SimpleWindow::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_SimpleWindow.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int SimpleWindow::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Simple Window
/*-------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 Mar 2021 16:00:04 IST
* File : qt_t002_ch2_simple_layout.cpp
* Title : Project to display simple layout
* Description : A Window that contain Horizantal and Vertical layouts. In each layout, different widgets
* are inserted
*------------------------------------------------------------------------------------------------------------*/
#include
#include "simplewindow.h"
SimpleWindow::SimpleWindow(QWidget *parent) :
QWidget(parent)
{
QLabel* noteLabel = new QLabel("Note:", this);
QTextEdit* noteEdit = new QTextEdit(this);
QPushButton* clearButton = new QPushButton("Clear", this);
QPushButton* saveButton = new QPushButton("Save", this);
/* outer horizontal-layout */
QVBoxLayout *outer = new QVBoxLayout;
outer->addWidget(noteLabel);
outer->addWidget(noteEdit);
/* nested inner layout */
QHBoxLayout *inner = new QHBoxLayout;
inner->addWidget(clearButton);
inner->addWidget(saveButton);
outer->addLayout(inner);
QSlider newr;
this->setLayout(outer);
}
Simple Layout Pro
SOURCES += main.cpp \
simplewindow.cpp
HEADERS += \
simplewindow.h
QT += widgets
QT Programming Example output:
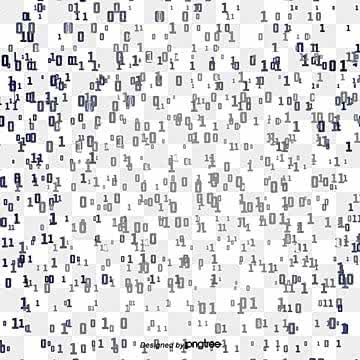

Brief:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project on container classes part 1
* Description : A demo code for container classes. qDebug is used to print the message.
* QStringList is used to print the strings
*------------------------------------------------------------------------------------------------------------*/
#include
bool operator<(const QPoint &p1, const QPoint &p2)
{
return ( p1.x() < p2.x() ||
(p1.x() == p2.x() && p1.y() < p2.y() ) );
}
uint qHash(const QPoint &p)
{
return qHash(p.x()) ^ qHash(p.y());
}
int main()
{
QMap<QPoint, int> map;
map.insert(QPoint(1, 2), 42);
int value = map.value(QPoint(1, 2));
Q_ASSERT(value == 42);
QHash<QPoint, int> hash;
hash.insert(QPoint(1, 1), 42);
QSet set;
set.insert(QPoint(1, 1));
// Check the location
QStringList strings;
strings << "abc" << "def" << "hij";
QMutableListIterator it(strings);
it.toBack();
qDebug() << "Just iterated over: " << it.previous();
it.insert("XXX");
qDebug() << "Next item after the insert is: " << it.previous();
it = QMutableListIterator(strings);
qDebug() << "Just iterated over: " << it.next();
it.insert("YYY");
qDebug() << "Next item after the insert is: " << it.next();
it = QMutableListIterator(strings);
while (it.hasNext())
qDebug() << it.next();
return 0;
}
Containers Pro
TEMPLATE = app SOURCES= main.cpp
QT Programming Example output:
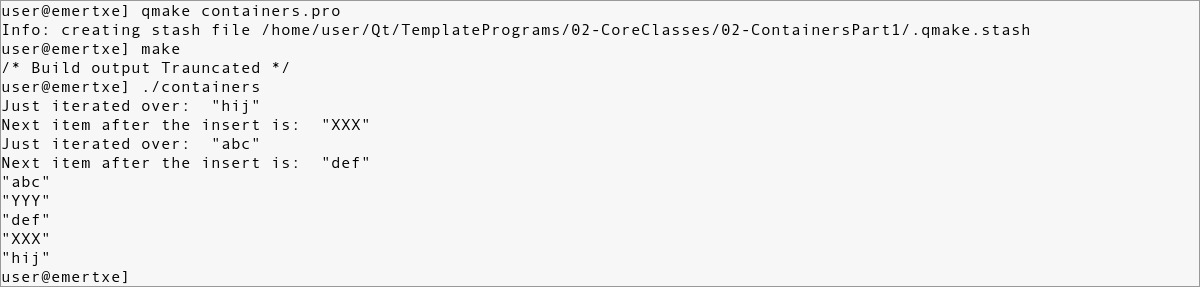
Brief:
A simple layout to display text editor with 2 buttons.
Main File:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project on container classes part 2
* Description : A demo code for container classes. qDebug is used to print the message.
* QList can be used to store integers or strings.
*-------------------------------------------------------------------------------------------------------------*/
#include
#include
#include
#include
#include
#include
#include
#include
int main()
{
/* QList
*/
{
QList list;
list << "one" << "two" << "three";
QString item1 = list[1]; // “two”
for(int i=0; i<list.count(); i++)
{
const QString &item2 = list.at(i);
}
int index = list.indexOf("two"); // returns 1
}
/* QMap
*/
{
QMap<QString, int> map;
map["Norway"] = 5; map["Italy"] = 48;
int value = map["France"]; // inserts key if not exists
if(map.contains("Norway"))
{
int value2 = map.value("Norway"); // recommended lookup
}
}
/* Iterators
*/
{
QList list;
list << "A" << "B" << "C" << "D";
QListIterator it(list);
while(it.hasNext())
{
qDebug() << it.next(); // A B C D
}
it.toBack(); // position after the last item
while(it.hasPrevious())
{
qDebug() << it.previous(); // D C B A
}
}
/* Modifying During Iteration
*/
{
QList list;
list << 1 << 2 << 3 << 4;
QMutableListIterator i(list);
while (i.hasNext())
{
if (i.next() % 2 != 0)
i.remove();
}
}
/* QMap iterator
* */
{
QMap<QString, QString> map;
map["Paris"] = "France";
map["Guatemala City"] = "Guatemala";
map["Mexico City"] = "Mexico";
map["Moscow"] = "Russia";
QMutableMapIterator<QString, QString> i(map);
while (i.hasNext())
{
if (i.next().key().endsWith("City"))
i.remove();
}
}
// map now "Paris", "Moscow"
return 0;
}
Containers Pro
SOURCES += \ main.cpp QT += widgets TEMPLATE = app
QT programming Example output:
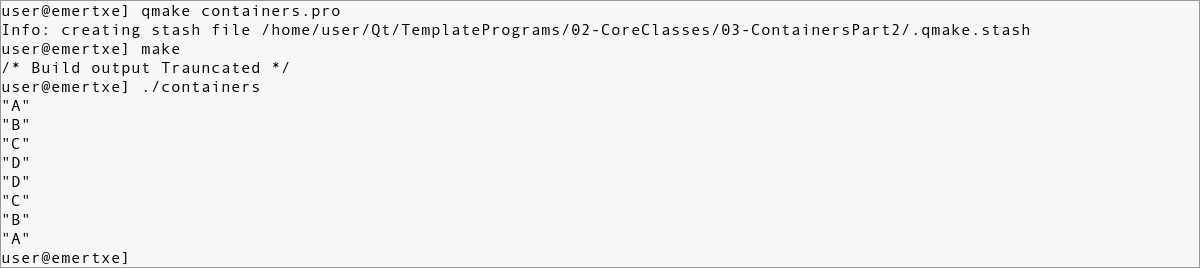
Brief:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project on Strings
* Description : A demo code to print strings in different methods.
*------------------------------------------------------------------------------------------------------------*/
#include
#include
#include
#include
int main(int argc, char *argv[])
{
/* Conversion constructor and assignment operators:
*/
QString str("abc");
str = "def";
qDebug() << str;
/* From a number using a static function:
*/
QString n = QString::number(1234);
qDebug() << n;
/* From a char pointer using a static function:
*/
QString text = QString::fromLatin1("Hello Qt");
qDebug() << text;
text = QString::fromUtf8("inputText");
qDebug() << text;
text = QString::fromLocal8Bit("cmdLineInput");
qDebug() << text;
text = QStringLiteral("Literal String");
qDebug() << text;
/* From char pointer with translations:
*/
text = QObject::tr("Hello Qt");
qDebug() << text;
/* Operators and right, left and mid justifications
* */
QString fileName = "MyFile";
fileName += ".txt";
qDebug() << fileName;
QString s = "apple";
QString t = s.leftJustified(8, '.');
qDebug() << t;
/* QString::arg()
*/
int i = 10;
int total = 99;
QString file = "MyFile.txt";
QString status = QObject::tr("Processing file %1 of %2: %3").arg(i).arg(total).arg(fileName);
qDebug() << status;
return 0;
}
Strings Pro
SOURCES += \ main.cpp QT += widgets TEMPLATE = app
QT programming Example output:
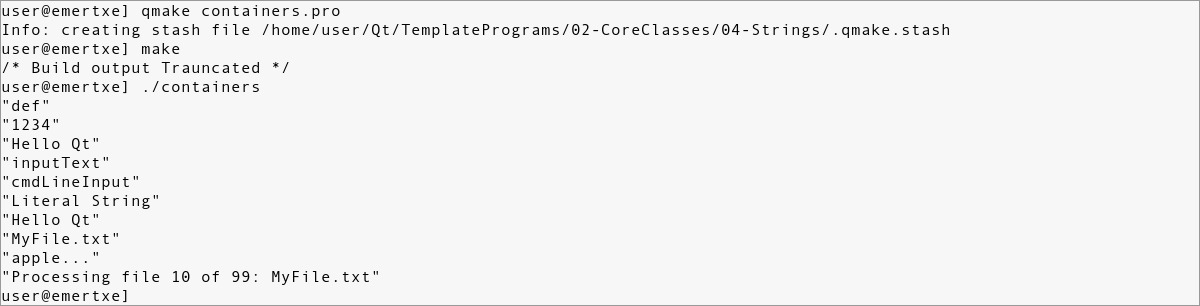
Chapter 3 : Widgets
Brief:
#ifndef WINDOW_H
#define WINDOW_H
#include
class window : public QWidget
{
Q_OBJECT
public:
explicit window(QWidget *parent = 0);
signals:
public slots:
protected:
void paintEvent(QPaintEvent *evnt);
};
#endif // WINDOW_H
Window File
/*-----------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : window.cpp
* Title : A demo code of drawing a cubic curve
* Description : In this code, the cubic curve is drawn using QPainterPath
*----------------------------------------------------------------------------------------------------------*/
#include
#include "window.h"
window::window(QWidget *parent) : QWidget(parent)
{
}
void window::paintEvent(QPaintEvent *evnt)
{
QPainterPath path;
//path.addRect(10,10,80,80);
path.cubicTo(100, 0, 50, 50, 100, 100);
path.moveTo(0,0);
path.cubicTo(0, 100, 50, 50, 100, 100);
QPainter painter(this);
painter.drawPoint(100, 0);
painter.drawPoint(50, 50);
painter.drawPoint(100, 100);
painter.drawPath(path);
}
Main File
/*-------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project on Text Widgets
* Description : A demo code for text widgets. A sample window which shows that we can read string as
* plain text or a text as a password or with highlighted color.
*-----------------------------------------------------------------------------------------------------------*/
#include
#include
int main(int argc, char *argv[])
{
QApplication app(argc,argv);
/* Window widget to show the text widgets */
QWidget *window = new QWidget;
/* Layout to arrange widgets in window */
QVBoxLayout *layout = new QVBoxLayout;
/* QLabel */
QLabel *label = new QLabel("Text", window);
/* You can set a image also in QLabel using QPixMap */
QPixmap pix("C:/Users/mkbij/OneDrive/Pictures/exmertxe_logo.png");
/* Setting pixmap to label */
label->setPixmap(pix);
/* Adding to label to layout */
layout->addWidget(label);
/* QLineEdit */
QLineEdit *user = new QLineEdit(window);
user->setText("User name");
QLineEdit *pass = new QLineEdit(window);
pass->setEchoMode(QLineEdit::Password);
/* Adding to layout */
layout->addWidget(user);
layout->addWidget(pass);
QTextEdit *text = new QTextEdit(window);
/* Adding text as plane text */
text->setPlainText("Hello");
/* Adding text as HTML */
text->append("
MOC Window File:
/**********************************************************************************************
** Meta object code from reading C++ file 'window.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
**********************************************************************************************/
#include "window.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'window.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_window_t {
QByteArrayData data[1];
char stringdata0[7];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_window_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_window_t qt_meta_stringdata_window = {
{
QT_MOC_LITERAL(0, 0, 6) // "window"
},
"window"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_window[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
0, 0, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
0 // eod
};
void window::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
Q_UNUSED(_o);
Q_UNUSED(_id);
Q_UNUSED(_c);
Q_UNUSED(_a);
}
QT_INIT_METAOBJECT const QMetaObject window::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_window.data,
qt_meta_data_window,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *window::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *window::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_window.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int window::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Text Widgets Pro File:
SOURCES += \
main.cpp \
window.cpp
QT = widgets
HEADERS += \
window.h
QT programming Example output:


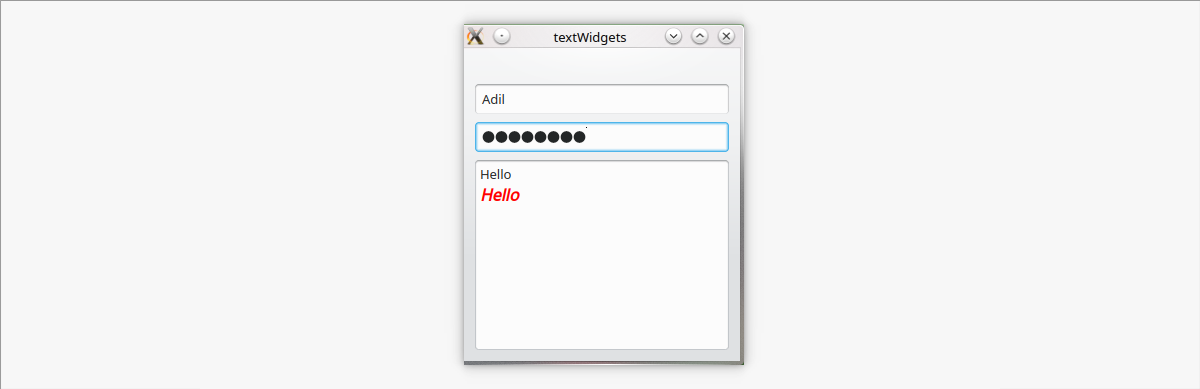
Brief:
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project on Item Widgets
* Description : A demo code for item widgets. A QComboBox is created to display dropdown menu
* If we select item and press the button, then respective item should be displayed
*-------------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char*argv[])
{
QApplication app(argc,argv);
app.setApplicationDisplayName("ComboBox");
/* combo box */
QWidget *window = new QWidget;
/* this layout to arrange one label and combobox */
QHBoxLayout *inner_one = new QHBoxLayout;
/* TO arrange pushbutton */
QHBoxLayout *inner_two = new QHBoxLayout;
/* To arrange inner layouts in vertical order */
QVBoxLayout *outer = new QVBoxLayout;
QComboBox *combo = new QComboBox(window);
QLabel *label = new QLabel("Items", window);
for(int i = 1; i < 4; i++) { combo->addItem("item "+QString::number(i));
}
QPushButton *button = new QPushButton("Which item", window);
/* TODO
* 1. Using signal and slot display current item in combo box when we press the button
* 2. Try Listwidget by your own */
inner_one->addWidget(label);
inner_one->addWidget(combo);
inner_two->addStretch(1);
inner_two->addWidget(button);
outer->addLayout(inner_one);
outer->addLayout(inner_two);
window->setLayout(outer);
window->show();
return app.exec();
}
Item Widgets Pro File:
SOURCES += \
main.cpp
QT = widgets
QT programming Example output:



Brief:
#ifndef QUOTEBUTTON_H
#define QUOTEBUTTON_H
#include
#include
class QuoteButton: public QPushButton
{
Q_OBJECT
public:
QuoteButton(const QString &text, QWidget *parent = 0);
signals:
void quote(const QString &);
protected slots:
void sendQuote();
private:
int m_quoteNo;
QStringList m_quotes;
};
#endif // QUOTEBUTTON_H
Quote Button File
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : quotebutton.cpp
* Title : Project to demonstrate signals and slots
* Description : A demo project to show custom signals. Multiple strings are stored using append(). When user
* clicks the quote button, new quote(next quote) should be displayed
*--------------------------------------------------------------------------------------------------------------*/
#include "quotebutton.h"
QuoteButton::QuoteButton(const QString &text, QWidget *parent)
: QPushButton(text, parent), m_quoteNo(-1)
{
// Quotes from Office Space!
m_quotes.append( "I'm a Michael Bolton fan. Could you play it for me?" );
m_quotes.append( "About your TPS reports..." );
m_quotes.append( "You will have to come in Saturday, will'ya?" );
m_quotes.append( "we're putting new coversheets on all the TPS reports *before* they go out now" );
m_quotes.append( "bear with me for just a second" );
m_quotes.append( "You see Bob, it's not that I'm lazy, it's that I just don't care." );
m_quotes.append( "No, not again." );
m_quotes.append( "I swear to God, one of these days..." );
m_quotes.append( "that's just a straight shooter with upper management written all over him" );
m_quotes.append( "Standard operating procedure." );
m_quotes.append( "I could set the building on fire." );
m_quotes.append( "Excuse me, I believe you have my stapler... " );
m_quotes.append( "I could put... I could put... strychnine in the guacamole." );
m_quotes.append( "What am I gonna do with 40 subscriptions to Vibe?" );
m_quotes.append( "Oh, and next Friday... is Hawaiian shirt day..." );
m_quotes.append( "You know, minimum security prison is no picnic." );
m_quotes.append( "I have people skills." );
m_quotes.append( "I can't believe what a bunch of nerds we are." );
m_quotes.append( "The ratio of people to cake is too big." );
connect( this, SIGNAL( clicked() ), this, SLOT( sendQuote() ) );
}
void QuoteButton::sendQuote()
{
m_quoteNo = (m_quoteNo + 1) % m_quotes.size();
emit quote( m_quotes[m_quoteNo] );
}
Main File
/*-----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate signals and slots
* Description : A demo project to show custom signals. Using signals and slots, communication is established
* between button and text editor.
*---------------------------------------------------------------------------------------------------------------*/
#include
#include "quotebutton.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *top = new QWidget;
top->setWindowTitle("Quotes from Office Space");
QuoteButton* button = new QuoteButton("Quote", top);
QTextEdit* edit = new QTextEdit(top);
QObject::connect(button, SIGNAL(quote(const QString &)), edit, SLOT(setText(const QString &)));
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(button);
layout->addWidget(edit);
top->setLayout(layout);
top->show();
return app.exec();
}
Quote Button Pro File:
HEADERS= quotebutton.h
SOURCES= main.cpp quotebutton.cpp
QT += widgets
QT programming Example output:




Brief:
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate Layouts
* Description : A demo project to demonstrate different types of layouts. Basically there are 4 types of
* layouts. Horizantal layout, Vertical layout, Form layout and Grid layout. In this demo code
* few buttons are created and the buttons are added in all these layouts.
*--------------------------------------------------------------------------------------------------------------*/
#include
class DemoWidget : public QWidget
{
public:
DemoWidget(QWidget *parent = 0)
: QWidget(parent)
{}
void addButtons(QLayout *layout)
{
layout->addWidget(new QPushButton("One"));
layout->addWidget(new QPushButton("Two"));
layout->addWidget(new QPushButton("Three"));
layout->addWidget(new QPushButton("Four"));
layout->addWidget(new QPushButton("Five"));
}
void createHBoxLayout()
{
QHBoxLayout *layout = new QHBoxLayout;
addButtons(layout);
setLayout(layout);
}
void createVBoxLayout()
{
QVBoxLayout *layout = new QVBoxLayout;
addButtons(layout);
setLayout(layout);
}
void createGridLayout()
{
QGridLayout *layout = new QGridLayout;
layout->addWidget(new QPushButton("One"), 0, 0);
layout->addWidget(new QPushButton("Two"), 0, 1);
layout->addWidget(new QPushButton("Three"), 1, 0, 1, 2);
setLayout(layout);
}
void createFormLayout()
{
QFormLayout *layout = new QFormLayout;
layout->addRow("One", new QPushButton("One"));
layout->addRow("Two", new QPushButton("Two"));
layout->addRow("Three", new QPushButton("Three"));
setLayout(layout);
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
DemoWidget row, col, grid, form;
row.createHBoxLayout();
col.createVBoxLayout();
grid.createGridLayout();
form.createFormLayout();
row.show();
col.show();
grid.show();
form.show();
return app.exec();
}
Layout Pro File:
TEMPLATE = app
TARGET = ex-layout
DEPENDPATH += .
INCLUDEPATH += .
# Input
SOURCES += main.cpp
QT += widgets
QT programming Example output:


Brief:
/*-----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate different types of buttons.
* Description : A demo project to demonstrate different types of buttons. In the following code radio buttos,
* checkbox buttons and pushbutton(bool) are shown
*---------------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *window = new QWidget;
/* Creating a layout for window */
QVBoxLayout *layout = new QVBoxLayout;
/* push button */
QPushButton *button = new QPushButton(window);
/* we can set an icon for button using QIcon */
button->setIcon(QIcon("C:/Users/mkbij/OneDrive/Pictures/qt.png"));
/* Resizing button & icon size */
button->setIconSize(QSize(128,128));
button->setFixedSize(QSize(128,128));
/* If you want a toggle button enable the checkable option*/
button->setCheckable(true);
/* Radio buttons */
QRadioButton *radio1 = new QRadioButton("Option 1",window);
QRadioButton *radio2 = new QRadioButton("Option 1",window);
layout->addWidget(radio1);
layout->addWidget(radio2);
/* Check box */
QCheckBox *check1 = new QCheckBox("Choice 1", window);
QCheckBox *check2 = new QCheckBox("Choice 2", window);
QCheckBox *check3 = new QCheckBox("Choice 3", window);
/* NOTE Group box Refer http://doc.qt.io/qt-5/qtwidgets-widgets-groupbox-example.html
* Adding check box widgets to group bo */
QVBoxLayout *vbox = new QVBoxLayout;
vbox->addWidget(check1);
vbox->addWidget(check2);
vbox->addWidget(check3);
QGroupBox *group = new QGroupBox;
group->setLayout(vbox);
/* Adding grop box to outer layout */
layout->addWidget(group);
/* Adding to layout */
layout->addWidget(button);
window->setLayout(layout);
window->show();
return app.exec();
Buttons Pro File:
SOURCES += \ main.cpp QT = widgets
QT programming Example output:



Brief:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate organizer widgets
* Description : A demo project on organizer widgets. This application contains 2 tabs, where each tab has
* text widget
*------------------------------------------------------------------------------------------------------------*/
#include
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *window = new QWidget;
QVBoxLayout *layout = new QVBoxLayout;
QTabWidget *mainTab = new QTabWidget(window);
mainTab->addTab(new QTextEdit, "Tab-1" );
mainTab->addTab(new QTextEdit, "Tab-2" );
/* Adding two text edits in two tabs
* NOTE : We can add any QWidget inherited objects to tabs
* TODO : Try to create a seperate class inherited from QWidget and try to add that class object to tabs
* The custom widget must have one text widget and two push buttons save and clear*/
layout->addWidget(mainTab);
window->setLayout(layout);
window->show();
return app.exec();
}
Organizer Widgets Pro File:
SOURCES += \
main.cpp
QT = widgets
QT programming Example output:




Brief:
A demo project to demonstrate different type of value widgets. Using slider or spin box we can update the value and display in progress bar. We need to use signals to connect the widgets.
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate value widgets.
* Description : A demo project to demonstrate different type of value widgets. Using slider or spin box
* we can update the value and display in progress bar. We need to use signals to connect the
* widgets.
*---------------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *window = new QWidget;
QVBoxLayout *layout = new QVBoxLayout;
/* Sliders */
QSlider *slider = new QSlider(Qt::Horizontal, window);
/* Setting range for slider */
slider->setRange(0,99);
/* Setting initial calue for slider */
slider->setValue(0);
/* Progress bar */
QProgressBar *progress = new QProgressBar(window);
progress->setRange(0,150);
progress->setValue(0);
progress->setFormat("%v - %p");
/* Spin box */
QSpinBox *spin = new QSpinBox(window);
spin->setRange(0,99);
spin->setValue(0);
spin->setSuffix(" INR");
/* Adding to layout */
layout->addWidget(slider);
layout->addStretch(1);
layout->addWidget(progress);
layout->addStretch(1);
layout->addWidget(spin);
window->setLayout(layout);
window->show();
/* TODO
* 1. Try to implement this in seperate class.
* 2. Using signals and slots connect slider and progress bar (When slider value changes, change progressbar)
* 3. Connect slider and spin box (When slider value changes, change spin box also).
* 4. connect spin box and slider (When spinr value changes, change slider also). */
return app.exec();
Value Widgets Pro File:
SOURCES += \
main.cpp
QT = widgets
QT programming Example output:




Chapter 4 : Object Communication
Brief:
/*-------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : Project to demonstrate connect function pointers
* Description : A demo project on connect(). In this application if we move the slider, then value of
* progress bar is changed.
*-----------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *window = new QWidget;
QSlider *slider = new QSlider(Qt::Horizontal);
slider->setRange(0, 100);
QProgressBar *bar = new QProgressBar;
/* This method will only work in Qt5, Its a modified version of SIGNAL & SLOT macros*/
QObject::connect(slider, &QSlider::valueChanged, bar, &QProgressBar::setValue);
/* slider and progress bar are connected, change will be propagated to progress bar */
slider->setValue(40);
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(slider);
layout->addWidget(bar);
window->setLayout(layout);
window->show();
return app.exec();
}
Connect Function Pointers Pro File:
TEMPLATE=app SOURCES = main.cpp QT += wid
QT programming Example output:



Brief:
/*-------------------------------------------------------------------------------------------------------------------- * Author : Emertxe (https://emertxe.com) * Date : Wed 08 May 2021 16:00:04 IST * File : main.cpp * Title : A demo project to connect two QObjects with non-member funtion * Description : In this application, based on the movement of the slider, progress bar is changed. * at the same time the current value of slider/progress bar will be printed using qDebug function. *-------------------------------------------------------------------------------------------------------------------*/ #include QProgressBar *bar; static void printValue(int value) { qDebug("Value is: %d", value); bar->setValue(value); } int main(int argc, char *argv[]) { QApplication app(argc, argv); QWidget *window = new QWidget; QSlider *slider = new QSlider(Qt::Horizontal); slider->setRange(0, 100); bar = new QProgressBar; QObject::connect( slider, &QSlider::valueChanged, &printValue ); // slider and progress bar are connected, change will be propagated to progress bar slider->setValue(40); QHBoxLayout *layout = new QHBoxLayout; layout->addWidget(slider); layout->addWidget(bar); window->setLayout(layout); window->show(); return app.exec(); }
Connect Non member Pro File:
TEMPLATE=app SOURCES = main.cpp QT += widgets
QT programming Example output:




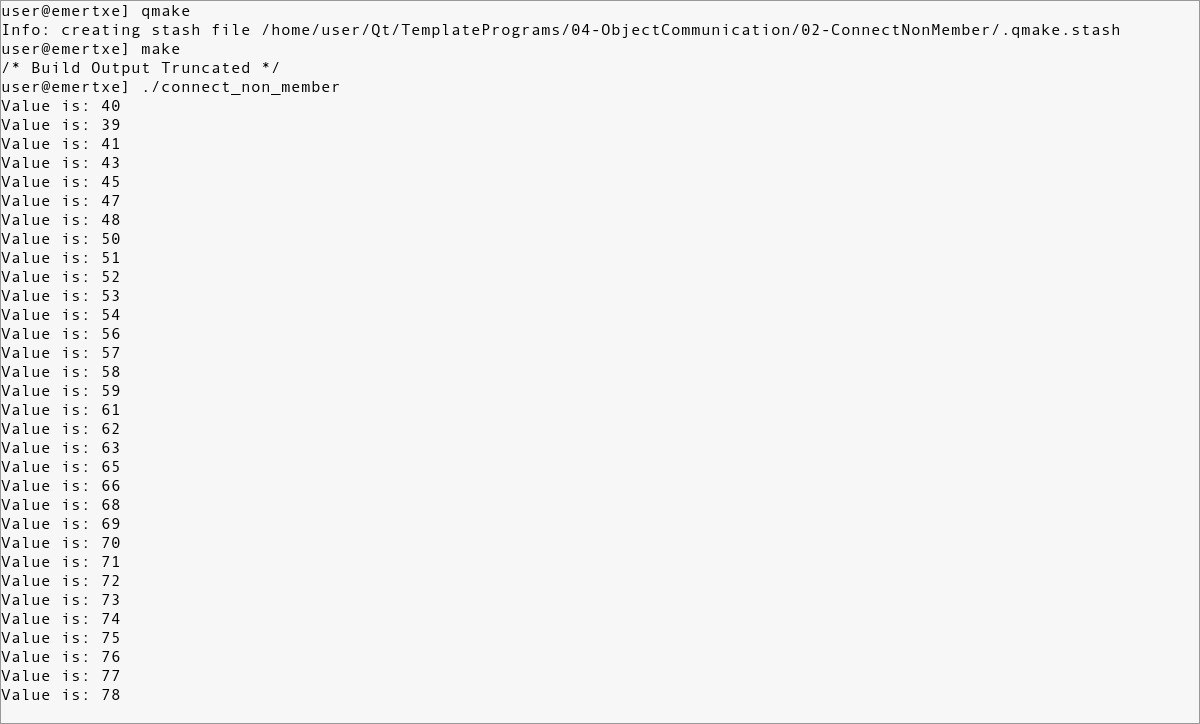
Brief:
/*-----------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo project to connect two Objects
* Description : In this application, based on the movement of the slider, progress bar is changed.
*--------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *window = new QWidget;
QSlider *slider = new QSlider(Qt::Horizontal);
slider->setRange(0, 100);
QProgressBar *bar = new QProgressBar;
QObject::connect(slider, SIGNAL(valueChanged(int)), bar, SLOT(setValue(int)));
// slider and progress bar are connected, change will be propagated to progress bar
slider->setValue(40);
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(slider);
layout->addWidget(bar);
window->setLayout(layout);
window->show();
return app.exec();
}
Connect Pro File:
TEMPLATE=app
SOURCES = main.cpp
QT += widgets
QT programming Example output:



Brief:
/****************************************************************************************************
*
* Discription : A demo project for custom slots
* Author : Biju
* Company : Emertxe info tech
*
***************************************************************************************************/
#ifndef STOPWATCH_H
#define STOPWATCH_H
#include
class StopWatch : public QLabel
{
Q_OBJECT
public:
StopWatch(QWidget *parent = 0);
protected slots:
void shot();
private:
int m_secs;
};
#endif
Stop Watch:
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : stopwatch.cpp
* Title : A demo project for custom slots
* Description : In this application, stop watch is created and time wll be displayed in secs starting
* from 0:00
*-------------------------------------------------------------------------------------------------------------*/
#include
#include "stopwatch.h"
StopWatch::StopWatch(QWidget *parent)
: QLabel(parent), m_secs(0)
{
setText("0:00");
QTimer *timer = new QTimer(this);
timer->start(1000);
connect(timer, SIGNAL(timeout()), this, SLOT(shot()));
}
void StopWatch::shot()
{
m_secs += 1;
QString str;
str.sprintf( "%d:%02d", m_secs / 60, m_secs % 60 );
setText( str );
}
MOC Stopwatch File:
/************************************************************************************************************
** Meta object code from reading C++ file 'stopwatch.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
************************************************************************************************************/
#include "stopwatch.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'stopwatch.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_StopWatch_t {
QByteArrayData data[3];
char stringdata0[16];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_StopWatch_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_StopWatch_t qt_meta_stringdata_StopWatch = {
{
QT_MOC_LITERAL(0, 0, 9), // "StopWatch"
QT_MOC_LITERAL(1, 10, 4), // "shot"
QT_MOC_LITERAL(2, 15, 0) // ""
},
"StopWatch\0shot\0"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_StopWatch[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
1, 14, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
// slots: name, argc, parameters, tag, flags
1, 0, 19, 2, 0x09 /* Protected */,
// slots: parameters
QMetaType::Void,
0 // eod
};
void StopWatch::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
if (_c == QMetaObject::InvokeMetaMethod) {
auto *_t = static_cast(_o);
Q_UNUSED(_t)
switch (_id) {
case 0: _t->shot(); break;
default: ;
}
}
Q_UNUSED(_a);
}
QT_INIT_METAOBJECT const QMetaObject StopWatch::staticMetaObject = { {
&QLabel::staticMetaObject,
qt_meta_stringdata_StopWatch.data,
qt_meta_data_StopWatch,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *StopWatch::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *StopWatch::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_StopWatch.stringdata0))
return static_cast<void*>(this);
return QLabel::qt_metacast(_clname);
}
int StopWatch::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QLabel::qt_metacall(_c, _id, _a);
if (_id < 0)
return _id;
if (_c == QMetaObject::InvokeMetaMethod) {
if (_id < 1)
qt_static_metacall(this, _c, _id, _a);
_id -= 1;
} else if (_c == QMetaObject::RegisterMethodArgumentMetaType) {
if (_id < 1)
*reinterpret_cast<int*>(_a[0]) = -1;
_id -= 1;
}
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Main File:
/*--------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo project for custom slots
* Description : In this application, stop watch is created and time wll be displayed in secs starting from 0:00
* using the StopWatch class we can create a stop watch
*------------------------------------------------------------------------------------------------------------------*/
#include
#include "stopwatch.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
StopWatch *watch = new StopWatch;
watch->show();
return app.exec();
}
Stop Watch Pro File:
HEADERS = stopwatch.h
SOURCES = main.cpp \
stopwatch.cpp
TEMPLATE = app
QT += widgets
Example output:

Brief:
/*************************************************************************************************
*
* Discription : A Demo code for event handling
* Author : Biju
* Company : Emertxe info tech
*
************************************************************************************************/
#ifndef WIDGET_H
#define WIDGET_H
#include
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = 0);
protected:
void closeEvent(QCloseEvent *event);
private:
bool windowShouldClose();
};
#endif // WIDGET_H
Widget File:
/*-------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for event handling
* Description : There are different categories of event handling, one of them is "close event". If user tries
* to close the application, then a pop-up message is displayed for confirmation. Based on the
* user i/p application will be closed or input will be ignored.
*-----------------------------------------------------------------------------------------------------------------*/
#include
#include "widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
setWindowTitle("Close Event [*]");
setWindowModified(true);
QLabel *label = new QLabel("Try to close the window.");
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(label, 0, Qt::AlignCenter);
setLayout(layout);
}
void Widget::closeEvent(QCloseEvent *event)
{
if (windowShouldClose())
event->accept();
else
event->ignore();
}
bool Widget::windowShouldClose()
{
if (!isWindowModified())
return true;
QMessageBox::StandardButton answer =
QMessageBox::question(
this,
"Close Window",
"Really close the window?",
QMessageBox::Yes | QMessageBox::No
);
return (answer == QMessageBox::Yes);
}
MOC Widget File:
/**************************************************************************************************************
** Meta object code from reading C++ file 'widget.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
**************************************************************************************************************/
#include "widget.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'widget.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_Widget_t {
QByteArrayData data[1];
char stringdata0[7];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_Widget_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_Widget_t qt_meta_stringdata_Widget = {
{
QT_MOC_LITERAL(0, 0, 6) // "Widget"
},
"Widget"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_Widget[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
0, 0, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
0 // eod
};
void Widget::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
Q_UNUSED(_o);
Q_UNUSED(_id);
Q_UNUSED(_c);
Q_UNUSED(_a);
}
QT_INIT_METAOBJECT const QMetaObject Widget::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_Widget.data,
qt_meta_data_Widget,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *Widget::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *Widget::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_Widget.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int Widget::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Main File:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for event handling
* Description : In this application, if user tries to close the window then pop-up message is displayed
* for confirmation. If user selects Yes, then application will be terminated.
*-----------------------------------------------------------------------------------------------------------*/
#include
#include "widget.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Widget window;
window.show();
return app.exec();
}
Stop Watch Pro File:
HEADERS = widget.h
SOURCES = main.cpp \
widget.cpp
QT += widgets
QT programming Example output:



Brief:
/*********************************************************************************************************
*
* Discription : A Demo code for event handling
* Author : Biju
* Company : Emertxe info tech
*
********************************************************************************************************/
#ifndef WIDGET_H
#define WIDGET_H
#include
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = 0);
void setLogAll(bool reduced);
protected:
// central event handler
bool event(QEvent *event);
// paint event
void paintEvent(QPaintEvent *event);
// input events
void keyPressEvent(QKeyEvent *event);
void keyReleaseEvent(QKeyEvent *event);
void mousePressEvent(QMouseEvent *event);
void mouseReleaseEvent(QMouseEvent *event);
void mouseDoubleClickEvent(QMouseEvent *event);
void mouseMoveEvent(QMouseEvent *event);
// focus events
void focusInEvent(QFocusEvent *event);
void focusOutEvent(QFocusEvent *event);
// geometry events
void resizeEvent(QResizeEvent *event);
void hideEvent(QHideEvent *event);
void showEvent(QShowEvent *event);
// other events
void closeEvent(QCloseEvent *event);
signals:
void notifyEvent(const QString &message, QEvent *event);
private:
bool m_logAll;
};
#endif
Event Log Window Header File:
/************************************************************************************************************
*
* Discription : A Demo code for event handling
* Author : Biju
* Company : Emertxe info tech
*
***********************************************************************************************************/
#ifndef EVENTLOGWINDOW_H
#define EVENTLOGWINDOW_H
#include
class EventLogWindow : public QWidget
{
Q_OBJECT
public:
explicit EventLogWindow(QWidget *parent = 0);
public slots:
void logEvent(const QString &message, QEvent *event);
private:
QTextEdit *m_edit;
};
#endif // EVENTLOGWINDOW_H
Widget File:
/*---------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : widget.cpp
* Title : A Demo code for event handling
* Description : In this window if user clicks, or focued or double clicked, or even if user moves
* out of the window, the event will be logged and displayed in another window
*-------------------------------------------------------------------------------------------------------*/
#include
#include "widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent), m_logAll(false)
{
setWindowTitle(tr("Widget Event Example"));
setFocusPolicy(Qt::StrongFocus);
}
bool Widget::event(QEvent *event)
{
if(m_logAll) {
qDebug() << "Handling all events in event()";
emit notifyEvent("event", event);
}
/* If you do not perform all the necessary work
* in your implementation of the virtual function,
* you may need to call the base class's implementation.*/
return QWidget::event(event);
}
void Widget::paintEvent(QPaintEvent *event)
{
emit notifyEvent("paintEvent", event);
QWidget::paintEvent(event);
}
// input events
void Widget::keyPressEvent(QKeyEvent *event)
{
emit notifyEvent("keyPressEvent", event);
QWidget::keyPressEvent(event);
}
void Widget::keyReleaseEvent(QKeyEvent *event)
{
emit notifyEvent("keyReleaseEvent", event);
QWidget::keyReleaseEvent(event);
}
void Widget::mousePressEvent(QMouseEvent *event)
{
emit notifyEvent("mousePressEvent", event);
QWidget::mousePressEvent(event);
// for context menus use contextMenuEvent(...)
}
void Widget::mouseReleaseEvent(QMouseEvent *event)
{
emit notifyEvent("mouseReleaseEvent", event);
QWidget::mouseReleaseEvent(event);
}
void Widget::mouseDoubleClickEvent(QMouseEvent *event)
{
emit notifyEvent("mouseDoubleClickEvent", event);
QWidget::mouseDoubleClickEvent(event);
}
void Widget::mouseMoveEvent(QMouseEvent *event)
{
emit notifyEvent("mouseMoveEvent", event);
QWidget::mouseMoveEvent(event);
}
// focus events
void Widget::focusInEvent(QFocusEvent *event)
{
emit notifyEvent("focusInEvent", event);
QWidget::focusInEvent(event);
}
void Widget::focusOutEvent(QFocusEvent *event)
{
emit notifyEvent("focusOutEvent", event);
QWidget::focusOutEvent(event);
}
// geometry events
void Widget::resizeEvent(QResizeEvent *event)
{
emit notifyEvent("resizeEvent", event);
QWidget::resizeEvent(event);
}
void Widget::hideEvent(QHideEvent *event)
{
emit notifyEvent("hideEvent", event);
QWidget::hideEvent(event);
}
void Widget::showEvent(QShowEvent *event)
{
emit notifyEvent("showEvent", event);
QWidget::showEvent(event);
}
// other events
void Widget::closeEvent(QCloseEvent *event)
{
emit notifyEvent("closeEvent", event);
QWidget::closeEvent(event);
}
void Widget::setLogAll(bool logAll)
{
m_logAll = logAll;
}
MOC Widget File:
/**************************************************************************************************************
** Meta object code from reading C++ file 'widget.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
**************************************************************************************************************/
#include "widget.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'widget.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_Widget_t {
QByteArrayData data[6];
char stringdata0[42];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_Widget_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_Widget_t qt_meta_stringdata_Widget = {
{
QT_MOC_LITERAL(0, 0, 6), // "Widget"
QT_MOC_LITERAL(1, 7, 11), // "notifyEvent"
QT_MOC_LITERAL(2, 19, 0), // ""
QT_MOC_LITERAL(3, 20, 7), // "message"
QT_MOC_LITERAL(4, 28, 7), // "QEvent*"
QT_MOC_LITERAL(5, 36, 5) // "event"
},
"Widget\0notifyEvent\0\0message\0QEvent*\0"
"event"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_Widget[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
1, 14, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
1, // signalCount
// signals: name, argc, parameters, tag, flags
1, 2, 19, 2, 0x06 /* Public */,
// signals: parameters
QMetaType::Void, QMetaType::QString, 0x80000000 | 4, 3, 5,
0 // eod
};
void Widget::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
if (_c == QMetaObject::InvokeMetaMethod) {
auto *_t = static_cast(_o);
Q_UNUSED(_t)
switch (_id) {
case 0: _t->notifyEvent((*reinterpret_cast< const QString(*)>(_a[1])),(*reinterpret_cast< QEvent*(*)>(_a[2]))); break;
default: ;
}
} else if (_c == QMetaObject::IndexOfMethod) {
int *result = reinterpret_cast(_a[0]);
{
using _t = void (Widget::*)(const QString & , QEvent * );
if (*reinterpret_cast<_t *>(_a[1]) == static_cast<_t>(&Widget::notifyEvent)) {
*result = 0;
return;
}
}
}
}
QT_INIT_METAOBJECT const QMetaObject Widget::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_Widget.data,
qt_meta_data_Widget,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *Widget::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *Widget::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_Widget.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int Widget::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
if (_id < 0)
return _id;
if (_c == QMetaObject::InvokeMetaMethod) {
if (_id < 1)
qt_static_metacall(this, _c, _id, _a);
_id -= 1;
} else if (_c == QMetaObject::RegisterMethodArgumentMetaType) {
if (_id < 1)
*reinterpret_cast<int*>(_a[0]) = -1;
_id -= 1;
}
return _id;
}
// SIGNAL 0
void Widget::notifyEvent(const QString & _t1, QEvent * _t2)
{
void *_a[] = { nullptr, const_cast<void*>(reinterpret_cast(&_t1)), const_cast<void*>(reinterpret_cast(&_t2)) };
QMetaObject::activate(this, &staticMetaObject, 0, _a);
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
MOC Event Log Window File:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : eventlogwindow.cpp
* Title : A Demo code for event handling
* Description : Based on the mouse event like clicked or moved or double clicked, the event will be
* displayed in this window
*--------------------------------------------------------------------------------------------------------------*/
#include "eventlogwindow.h"
EventLogWindow::EventLogWindow(QWidget *parent)
: QWidget(parent)
{
setWindowTitle("Event Logger");
m_edit = new QTextEdit;
m_edit->setFontFamily("Courier");
m_edit->setReadOnly(true);
QPushButton* clear = new QPushButton("Clear");
connect(clear, SIGNAL(clicked()), m_edit, SLOT(clear()));
QVBoxLayout* layout = new QVBoxLayout;
layout->setMargin(0);
layout->addWidget(m_edit);
layout->addWidget(clear);
setLayout(layout);
}
void EventLogWindow::logEvent(const QString &message, QEvent *event)
{
QString out;
QDebug(&out) << message.leftJustified(24, '.') << event; m_edit->append(out);
}
Main File:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for event handling
* Description : In this application, different type of events are notified and the respective will be
* noted in text editor. One window is used to generate the event and another windowto log
* in the Text editor
*-------------------------------------------------------------------------------------------------------------*/
#include
#include "widget.h"
#include "eventlogwindow.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
EventLogWindow logger;
logger.resize(640, 240);
logger.move(40, 0);
logger.show();
Widget window;
window.setLogAll(false); // does not log event(...) calls
window.resize(240, 320);
window.move(40, 320);
window.show();
QObject::connect(&window, SIGNAL(notifyEvent(QString, QEvent *)), &logger, SLOT(logEvent(QString, QEvent *)));
return app.exec();
}
All Events Pro File:
HEADERS = widget.h \
eventlogwindow.h
SOURCES = main.cpp \
widget.cpp \
eventlogwindow.cpp
QT += widgets
QT programming Example output:



Brief:
#ifndef EVENTTEST_H
#define EVENTTEST_H
#include
class eventtest:public QWidget
{
Q_OBJECT
public:
eventtest();
protected:
void mousePressEvent(QMouseEvent *event);
signals:
void clicked(QString);
};
#endif // EVENTTEST_H
MOC Event Test File:
/**********************************************************************************************************
** Meta object code from reading C++ file 'eventtest.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
*********************************************************************************************************/
#include "eventtest.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'eventtest.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_eventtest_t {
QByteArrayData data[3];
char stringdata0[19];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_eventtest_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_eventtest_t qt_meta_stringdata_eventtest = {
{
QT_MOC_LITERAL(0, 0, 9), // "eventtest"
QT_MOC_LITERAL(1, 10, 7), // "clicked"
QT_MOC_LITERAL(2, 18, 0) // ""
},
"eventtest\0clicked\0"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_eventtest[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
1, 14, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
1, // signalCount
// signals: name, argc, parameters, tag, flags
1, 1, 19, 2, 0x06 /* Public */,
// signals: parameters
QMetaType::Void, QMetaType::QString, 2,
0 // eod
};
void eventtest::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
if (_c == QMetaObject::InvokeMetaMethod) {
auto *_t = static_cast(_o);
Q_UNUSED(_t)
switch (_id) {
case 0: _t->clicked((*reinterpret_cast< QString(*)>(_a[1]))); break;
default: ;
}
} else if (_c == QMetaObject::IndexOfMethod) {
int *result = reinterpret_cast(_a[0]);
{
using _t = void (eventtest::*)(QString );
if (*reinterpret_cast<_t *>(_a[1]) == static_cast<_t>(&eventtest::clicked)) {
*result = 0;
return;
}
}
}
}
QT_INIT_METAOBJECT const QMetaObject eventtest::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_eventtest.data,
qt_meta_data_eventtest,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *eventtest::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *eventtest::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_eventtest.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int eventtest::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
if (_id < 0)
return _id;
if (_c == QMetaObject::InvokeMetaMethod) {
if (_id < 1)
qt_static_metacall(this, _c, _id, _a);
_id -= 1;
} else if (_c == QMetaObject::RegisterMethodArgumentMetaType) {
if (_id < 1)
*reinterpret_cast<int*>(_a[0]) = -1;
_id -= 1;
}
return _id;
}
// SIGNAL 0
void eventtest::clicked(QString _t1)
{
void *_a[] = { nullptr, const_cast<void*>(reinterpret_cast(&_t1)) };
QMetaObject::activate(this, &staticMetaObject, 0, _a);
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Event Test File:
/*-------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for mouse event
* Description : In this application, it displays the message about which mouse button is presed, either, left
* middle or right. This message is displayed using QMessageBox
*-----------------------------------------------------------------------------------------------------------------*/
#include "eventtest.h"
#include
eventtest::eventtest()
{
QLabel *label = new QLabel(this);
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(label);
setLayout(layout);
connect(this,SIGNAL(clicked(QString)), label, SLOT(setText(QString)));
}
void eventtest::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::RightButton)
{
QMessageBox::information(this, "Mouse event", "Right button pressed",QMessageBox::Ok);
emit clicked("Right Button");
}
else if(event->button() == Qt::LeftButton)
{
QMessageBox::information(this, "Mouse event", "Left button pressed",QMessageBox::Ok);
emit clicked("Left Button");
}
else
{
QMessageBox::information(this, "Mouse event", "Mid button pressed",QMessageBox::Ok);
emit clicked("Middle Button");
}
}
Main File:
/*--------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for mouse event
* Description : In this application, it displays the message about which mouse button is presed, either, left
* middle or right
*-----------------------------------------------------------------------------------------------------------------*/
#include
#include "eventtest.h"
int main(int argc, char **argv)
{
QApplication a(argc, argv);
eventtest myevent;
myevent.resize(150,150);
myevent.show();
return a.exec();
}
Mouse Events Pro File:
SOURCES += \
main.cpp \
eventtest.cpp
QT += widgets
TEMPLATE = app
HEADERS += \
eventtest.h
QT programming Example output:

Chapter 5 : Painting and Styling
Brief:
/*------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : eventtest.cpp
* Title : A Example code for understanding QPainter
* Description : In this application, it displays 3 rectangles which is filled with different colors.
* If we alter size of the application, size of the rectangles will not be affected
*----------------------------------------------------------------------------------------------------------*/
#include
class Test : public QWidget
{
public:
/* This override function will call automatically when a this object creats */
virtual void paintEvent(QPaintEvent *)
{
QPainter p(this);
p.setBrush(Qt::black);
/* QRectF(qreal x, qreal y, qreal width, qreal height) */
p.drawRect(QRectF(2.0, 2.0, 90.0, 90.0));
p.setBrush(Qt::red);
p.drawRect(QRectF(2.0, 102.0, 90.0, 90.0));
/* Giving more smooth effect in edges */
p.setRenderHint(QPainter::Antialiasing);
p.setBrush(Qt::blue);
p.drawRect(QRectF(2.0, 202.0, 90.0, 90.0));
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Test test;
test.resize(150, 400);
test.show();
return app.exec();
}
Rectangle Outline Pro File:
QT += widgets
TEMPLATE = app
SOURCES= main.cpp
CONFIG += debug
QT Programming Example output:

Brief:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code for gradient in painter
* Description : In this application, three different gradients are shown. If the size of the application
* changes, then size of gradients are also modified
*------------------------------------------------------------------------------------------------------------*/
#include
class Test : public QWidget
{
public:
Test(QWidget *parent = 0)
: QWidget(parent)
{}
protected:
void paintEvent(QPaintEvent *)
{
/* obtaining the current width and heght of window */
int w = width();
int h = height()/3;
QPainter painter(this);
{ /* A linear gradient with adjusting geometry according to current window size */
QLinearGradient gradient(5, 5, w-10, h-10);
/* Starting with a colour Red */
gradient.setColorAt(0, Qt::red);
gradient.setColorAt(0.5, Qt::green);
gradient.setColorAt(1, Qt::blue);
/* setting gradiant as a brush in painter */
painter.setBrush(gradient);
/* Using gradient painting a rectangle */
painter.drawRect(5, 5, w-10, h-10 );
}
{
QRadialGradient gradient(w/2+5, h+h/2+5, w/2, w/3, h+h/3);
gradient.setColorAt(0, Qt::white);
gradient.setColorAt(0.5, Qt::green);
gradient.setColorAt(1, Qt::black);
painter.setBrush(gradient);
painter.drawEllipse(5, h+5, w-10, h-10);
}
{
QConicalGradient gradient(2*w/3, 2*h+h/3, 0);
gradient.setColorAt(0, Qt::black);
gradient.setColorAt(0.4, Qt::green);
gradient.setColorAt(0.6, Qt::white);
gradient.setColorAt(1, Qt::black);
painter.setBrush(gradient);
painter.drawEllipse(5, 2*h+5, w-10, h-10);
}
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Test test;
test.resize(100, 300);
test.show();
return app.exec();
}
Gradients Pro File:
QT += widgets
SOURCES+=main.cpp
QT programming Example output:
Brief:
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A Demo code to print Hello world using Linear gradients
* Description : In this application, Hello world message is printed using Linear gradients
*--------------------------------------------------------------------------------------------------------------*/
#include
class Test : public QWidget
{
public:
Test(QWidget *parent = 0)
: QWidget(parent)
{
/* Setting window size according to word length and height */
QFont fnt = font();
fnt.setPixelSize(100);
QFontMetrics fm(fnt);
/* To get the size of a font */
setFixedSize(fm.width("Hello World"), fm.height());
}
protected:
void paintEvent(QPaintEvent *)
{
QPainter painter(this);
QFont font = painter.font();
font.setPixelSize(100);
painter.setFont(font);
QFontMetrics fm(font);
/* Setting a gradient using size of font */
QLinearGradient gradient(0, 0, fm.width("Hello World"), fm.height());
gradient.setColorAt(0, Qt::red);
gradient.setColorAt(0.5, Qt::green);
gradient.setColorAt(1, Qt::blue);
QBrush b(gradient);
/* setting gradiant brush to pen */
QPen pen(b,0);
pen.setStyle(Qt::SolidLine);
painter.setPen(pen);
painter.drawText(0, 100, "Hello World");
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Test test;
test.show();
return app.exec();
}
Pain with Brush Pro File:
QT = widgets
TEMPLATE = app
SOURCES= main.cpp
QT programming Example output:

Brief:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code for drawing figures
* Description : In this application, differnt figures are drawn by using differnet functions of QPainter
* class
*-------------------------------------------------------------------------------------------------------------*/
#include
class DrawingBoard : public QWidget
{
public:
QSize sizeHint() const {
return QSize(640, 490);
}
protected:
void paintEvent(QPaintEvent *)
{
QPainter p(this);
p.translate(20, 20);
QPolygon points;
points << QPoint(10, 10) << QPoint(30, 90) << QPoint(70, 90) << QPoint(90, 10); p.setRenderHint(QPainter::Antialiasing); p.setRenderHint(QPainter::HighQualityAntialiasing); p.setRenderHint(QPainter::TextAntialiasing); p.setRenderHint(QPainter::SmoothPixmapTransform); QFont font = p.font(); font.setPixelSize(10); p.setFont(font); p.setPen(QPen(Qt::red, 2)); p.setBrush(Qt::yellow); drawBox(&p); p.drawPoint(points[0]); drawTitle(&p, "drawPoint()"); nextColumn(&p); drawBox(&p); p.drawLine(points[0], points[1]); drawTitle(&p, "drawLine()"); nextColumn(&p); drawBox(&p); p.drawPolyline(points); drawTitle(&p, "drawPolyline()"); nextColumn(&p); drawBox(&p); p.drawPoints(points); drawTitle(&p, "drawPoints()"); nextColumn(&p); drawBox(&p); p.drawLines(points); drawTitle(&p, "drawLines()"); nextColumn(&p); drawBox(&p); p.drawPolygon(points); drawTitle(&p, "drawPolygon()"); nextRow(&p); drawBox(&p); p.drawRect(QRect(10, 10, 80, 80)); drawTitle(&p, "drawRect()"); nextColumn(&p); drawBox(&p); p.drawRoundedRect(QRect(10, 10, 80, 80), 8, 8); drawTitle(&p, "drawRoundedRect()"); nextColumn(&p); drawBox(&p); p.drawEllipse(QRect(10, 20, 80, 60)); drawTitle(&p, "drawEllipse()"); nextColumn(&p); drawBox(&p); p.drawArc(QRect(10, 20, 80, 60), 16 * 45, 16 * 135); drawTitle(&p, "drawArc()"); nextColumn(&p); drawBox(&p); p.drawChord(QRect(10, 20, 80, 60), 16 * 45, 16 *135); drawTitle(&p, "drawChord()"); nextColumn(&p); drawBox(&p); p.drawPie(QRect(10, 20, 80, 60), 16 * 45, 16 * 135); drawTitle(&p, "drawPie()"); nextRow(&p); drawBox(&p); p.drawRect(QRect(10, 20, 80, 60)); p.drawText(QRect(10, 20, 80, 60), Qt::AlignCenter, "Qt"); drawTitle(&p, "drawText()"); nextColumn(&p); drawBox(&p); p.drawPixmap(QRect(10, 10, 80, 80), QPixmap("image.jpg")); drawTitle(&p, "drawPixmap()"); nextColumn(&p); drawBox(&p); QPainterPath path; path.addEllipse(QRectF(10, 20, 80, 60)); path.addRect(QRect(10, 10, 80, 80)); p.drawPath(path); drawTitle(&p, "drawPath()"); } void drawTitle(QPainter *p, const QString &text) { p->save();
p->setPen(Qt::blue);
p->drawText(QRect(0, 100, 100, 50), text, QTextOption(Qt::AlignCenter));
p->restore();
}
void nextColumn(QPainter *p)
{
p->translate(100, 0);
}
void nextRow(QPainter *p)
{
p->translate(-p->transform().dx() + 20, 150);
}
void drawBox(QPainter *p)
{
p->save();
p->setPen(Qt::blue);
p->setBrush(Qt::NoBrush);
p->drawRect(0, 0, 100, 100);
p->restore();
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
DrawingBoard board;
board.show();
return app.exec();
}
Figures Pro File:
QT += widgets
SOURCES += main.cpp
CONFIG -= app_bundle
QT programming Example output:

Brief:
Main File:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code for cordinate transform
* Description : In this application, how to move or rotate the coordinates are shown
*------------------------------------------------------------------------------------------------------------*/
#include
class DrawingBoard : public QWidget
{
public:
QSize sizeHint() const {
return QSize(320, 490);
}
protected:
void paintEvent(QPaintEvent *)
{
QPainter p(this);
p.translate(20, 20);
QColor red(Qt::red);
QRect box(10, 10, 80, 80);
QRect box2 = box.adjusted(10, 10, -10, -10);
QPolygon points;
points << QPoint(10, 10) << QPoint(30, 90) << QPoint(70, 90) << QPoint(90, 10); p.setRenderHint(QPainter::Antialiasing); p.setRenderHint(QPainter::TextAntialiasing); QFont font = p.font(); font.setPixelSize(10); p.setFont(font); p.setPen(Qt::red); p.setBrush(Qt::yellow); drawBox(&p); p.drawLine(points[0], points[1]); p.translate(20, 0); p.drawLine(points[0], points[1]); p.translate(-20, 0); drawTitle(&p, "translate"); nextColumn(&p); drawBox(&p); p.save(); p.setPen(red.lighter(150)); p.drawRect(box); p.scale(3, 3); // p.shear(2,5); p.restore(); drawCoord(&p, QPoint(0,0)); drawTitle(&p, "shear"); nextRow(&p); drawBox(&p); p.save(); p.setPen(red.lighter(125)); p.drawRect(box); p.scale(.5, .5); p.setPen(red.lighter(150)); p.drawRect(box); p.scale(2, 2); p.restore(); drawCoord(&p, QPoint(0,0)); drawTitle(&p, "scale"); nextColumn(&p); drawBox(&p); p.save(); p.drawRect(box2); p.translate(box2.center()); p.setPen(QPen(red.lighter(125), 1, Qt::DashLine)); p.setBrush(Qt::NoBrush); p.drawRect(box2); p.scale(.5, .5); p.setPen(QPen(red.lighter(150), 1, Qt::DashLine)); p.drawRect(box2); p.translate(-box2.center()); p.setPen(red); p.drawRect(box2); p.restore(); drawCoord(&p, box2.center()); drawTitle(&p, "scale center"); nextRow(&p); drawBox(&p); p.save(); p.drawRect(box2); p.rotate(15); p.drawRect(box2); p.restore(); drawCoord(&p, QPoint(0, 0)); drawTitle(&p, "rotate"); nextColumn(&p); drawBox(&p); p.save(); p.drawRect(box2); p.translate(box2.center()); p.rotate(15); p.translate(-box2.center()); p.drawRect(box2); p.restore(); drawCoord(&p, box2.center()); drawTitle(&p, "rotate center"); } void drawTitle(QPainter *p, const QString &text) { p->save();
p->setPen(Qt::blue);
p->drawText(QRect(0, 100, 100, 50), text, QTextOption(Qt::AlignCenter));
p->restore();
}
void nextColumn(QPainter *p) {
p->translate(100, 0);
}
void nextRow(QPainter *p) {
p->translate(-p->transform().dx() + 20, 150);
}
void drawBox(QPainter *p)
{
p->save();
p->setPen(QColor(0, 0, 255, 64));
p->setBrush(Qt::NoBrush);
p->drawRect(0, 0, 100, 100);
p->restore();
}
void drawCoord(QPainter *p, QPoint c)
{
p->save();
p->setPen(Qt::DashLine);
p->drawLine(0, c.y(), 100, c.y());
p->drawLine(c.x(), 0, c.x(), 100);
p->restore();
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
DrawingBoard board;
board.setFixedSize(300,500);
board.show();
return app.exec();
}
Transform Pro File:
QT += widgets
SOURCES += main.cpp
QT programming Example output:

Brief:
/*------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of QPainterPath
* Description : Using pen we can draw the leaf(outer part) and using Brush we can fill it(inner part)
*----------------------------------------------------------------------------------------------------------*/
#include
#include
#include
class Canvas : public QWidget
{
public:
virtual void paintEvent(QPaintEvent *)
{
QPainterPath path;
path.moveTo(0, 0);
path.cubicTo(99, 0, 50, 50, 99, 99);
path.cubicTo(0, 99, 50, 50, 0, 0);
QPainter painter(this);
painter.setPen(QPen(QColor(79, 106, 25), 1, Qt::SolidLine, Qt::FlatCap, Qt::MiterJoin));
painter.setBrush(QColor(122, 163, 39));
painter.drawPath(path);
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Canvas board;
board.resize(100, 100);
board.show();
return a.exec();
}
Painter Path Pro File:
#-------------------------------------------------
#
# Project created by QtCreator 2017-01-09T17:13:45
#
#-------------------------------------------------
QT += core gui
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
TARGET = painterpath
TEMPLATE = app
SOURCES += main.cpp
QT programming Example output:


Brief:
/*---------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of creting window using Form
* Description : In this code, all the widgets are added in th form. Based on th input, style of form will
* be modified.
*-------------------------------------------------------------------------------------------------------------*/
#include
#include
#include "form.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
form widget;
widget.show();
qApp->setStyle(QStyleFactory::create("windows"));
return app.exec();
}
Form File:
/*--------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of creting window using Form
* Description : In this code, connection is established between Combo box and application, based on the
* option in combo box, style of the form/Grid is modified.
*------------------------------------------------------------------------------------------------------------*/
#include "form.h"
#include "ui_form.h"
#include
#include
form::form(QWidget *parent) : QWidget(parent)
{
Ui_Form *ui = new Ui_Form;
ui->setupUi(this);
/* Globally changing stylesheet of all QLineEdit objects */
qApp->setStyleSheet("QLineEdit { background: red; color: white; } QPushButton {background: green; } ");
/* Only change for paticular object */
ui->age->setStyleSheet("QLineEdit {background: blue; }");
connect(ui->style, SIGNAL(activated(int)), this, SLOT(changeStyle(int)));
/* Getting all possble style keys */
qDebug() <<QStyleFactory::keys(); ui->pushButton->setCheckable(true);
}
void form::changeStyle(int which)
{
switch (which)
{
case 0: qApp->setStyle(QStyleFactory::create("windows")); break;
case 1: qApp->setStyle(QStyleFactory::create("fusion")); break;
case 2: qApp->setStyle(QStyleFactory::create("WindowsXP")); break;
}
}
MOC Form File:
/**************************************************************************************************************
** Meta object code from reading C++ file 'form.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
**************************************************************************************************************/
#include "form.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'form.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_form_t {
QByteArrayData data[4];
char stringdata0[24];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_form_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_form_t qt_meta_stringdata_form = {
{
QT_MOC_LITERAL(0, 0, 4), // "form"
QT_MOC_LITERAL(1, 5, 11), // "changeStyle"
QT_MOC_LITERAL(2, 17, 0), // ""
QT_MOC_LITERAL(3, 18, 5) // "which"
},
"form\0changeStyle\0\0which"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_form[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
1, 14, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
// slots: name, argc, parameters, tag, flags
1, 1, 19, 2, 0x0a /* Public */,
// slots: parameters
QMetaType::Void, QMetaType::Int, 3,
0 // eod
};
void form::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
if (_c == QMetaObject::InvokeMetaMethod) {
auto *_t = static_cast
Form Header File:
#ifndef FORM_H
#define FORM_H
#include
class form : public QWidget
{
Q_OBJECT
public:
explicit form(QWidget *parent = 0);
signals:
public slots:
void changeStyle(int which);
};
#endif // FORM_H
Simple Qss Pro File:
QT += core gui widgets
SOURCES= main.cpp \
form.cpp
FORMS = form.ui
TEMPLATE = app
HEADERS += \
form.h
QT programming Example output:

Brief:
Main File:
/*----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of qsscascading
* Description : In this code, it's demonstrated that we can add style to the widgets
*--------------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget *top = new QWidget;
QHBoxLayout *layout = new QHBoxLayout(top);
QPushButton *button = new QPushButton("Hello");
layout->addWidget(button);
top->show();
QPushButton *button2 = new QPushButton("Hello 2");
button2->show();
qApp->setStyleSheet("* { color:red; }");
top->setStyleSheet("QPushButton { color:blue; }");
button->setStyleSheet("* { color:green; }");
// Notice how the color is used from button's style rather than from its parent
// even though the parent has a more specific selector.
return app.exec();
}
Qss Cascading Pro File:
QT += widgets
SOURCES= main.cpp
QT programming Example output:


Brief:
In this window, 4 buttons are added. We can style border of each button with different colors
Main File:
/*-------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code on qssconflict
* Description : In this window, 4 buttons are added. We can style border of each button with different colors
*-----------------------------------------------------------------------------------------------------------------*/
#include
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QDialog *dialog = new QDialog;
QHBoxLayout *layout = new QHBoxLayout(dialog);
QPushButton *button1 = new QPushButton("Test 1");
QPushButton *button2 = new QPushButton("Test 2");
QPushButton *button3 = new QPushButton("Test 3");
button3->setObjectName("button");
QPushButton *button4 = new QPushButton("Test 4");
layout->addWidget(button1);
layout->addWidget(button2);
layout->addWidget(button3);
layout->addWidget(button4);
dialog->show();
// First lets check that we actually are capable of setting colors on each side
// We will use green for win, and red for loose in the rest of the example.
const char *sheet1 =
"* {"
" border-width: 10px;"
" border-style: solid;"
"}\n"
"* {"
" border-top-color: green;"
"}\n"
"* {"
" border-bottom-color: blue;"
"}\n"
"* {"
" border-left-color: yellow;"
"}\n"
"* {"
" border-right-color: cyan;"
"}";
const char *sheet2 =
"* {"
" border-width: 10px;"
" border-style: solid;"
"}\n"
// A type selector wins over a universal selector
"* {"
" border-top-color: red;"
"}\n"
"QPushButton {"
" border-top-color: green;"
"}\n"
// Verify that the order does not matter
"QPushButton {"
" border-bottom-color: green;"
"}\n"
"* {"
" border-bottom-color: red;"
"}\n"
// Two classes in a selector wins over one
"QPushButton {"
" border-left-color: red;"
"}\n"
"QDialog QPushButton {"
" border-left-color: green;"
"}\n"
// Verify that the order does not matter
"QDialog QPushButton {"
" border-right-color: green;"
"}\n"
"QPushButton {"
" border-right-color: red;"
"}";
const char *sheet3 =
"* {"
" border-width: 10px;"
" border-style: solid;"
"}\n"
// Check that property specication wins over no specification
"QPushButton[enabled=\"true\"] {"
" border-top-color: green;"
"}\n"
"QPushButton {"
" border-top-color: red;"
"}\n"
// Verify that the order does not matter
"QPushButton {"
" border-bottom-color: red;"
"}\n"
"QPushButton[enabled=\"true\"] {"
" border-bottom-color: green;"
"}\n"
// Object name wins over property specification
"QPushButton#button {"
" border-left-color: green;"
"}\n"
"QPushButton[enabled=\"true\"] {"
" border-left-color: red;"
"}\n"
// Verify that the order does not matter
"QPushButton[enabled=\"true\"] {"
" border-right-color: red;"
"}\n"
"QPushButton#button {"
" border-right-color: green;"
"}";
const char *sheet4 =
"* {"
" border-width: 10px;"
" border-style: solid;"
"}\n"
// More properties win over fewer
"QPushButton[enabled=\"true\"][flat=\"false\"] {"
" border-top-color: green;"
"}\n"
"QPushButton[enabled=\"true\"] {"
" border-top-color: red;"
"}\n"
// Verify that the order does not matter
"QPushButton[enabled=\"true\"] {"
" border-bottom-color: red;"
"}\n"
"QPushButton[enabled=\"true\"][flat=\"false\"] {"
" border-bottom-color: green;"
"}\n"
// Here we have two with same score, the later wins
"QPushButton[enabled=\"true\"] {"
" border-left-color: red;"
"}\n"
"QPushButton[flat=\"false\"] {"
" border-left-color: green;"
"}\n"
// The same two lines reordered, still the later wins.
"QPushButton[flat=\"false\"] {"
" border-right-color: red;"
"}\n"
"QPushButton[enabled=\"true\"] {"
" border-right-color: green;"
"}";
button1->setStyleSheet(sheet1);
button2->setStyleSheet(sheet2);
button3->setStyleSheet(sheet3);
button4->setStyleSheet(sheet4);
return app.exec();
}
Qss Conflict Pro File:
QT += widgets
SOURCES= main.cpp
QT programming Example output:


Brief:
Main File:
/*------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of drawing a cubic curve
* Description : In this code, the cubic curve is drawn using QPainterPath
*----------------------------------------------------------------------------------------------------------------*/
#include
#include "window.h"
int main(int argc, char *argv[])
{
QApplication app(argc,argv);
window *board = new window;
board->resize(100,100);
board->show();
return app.exec();
}
Window File:
/*-------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : window.cpp
* Title : A demo code of drawing a cubic curve
* Description : In this code, the cubic curve is drawn using QPainterPath
*------------------------------------------------------------------------------------------------------------------*/
#include
#include "window.h"
window::window(QWidget *parent) : QWidget(parent)
{
}
void window::paintEvent(QPaintEvent *evnt)
{
QPainterPath path;
//path.addRect(10,10,80,80);
path.cubicTo(100, 0, 50, 50, 100, 100);
path.moveTo(0,0);
path.cubicTo(0, 100, 50, 50, 100, 100);
QPainter painter(this);
painter.drawPoint(100, 0);
painter.drawPoint(50, 50);
painter.drawPoint(100, 100);
painter.drawPath(path);
}
MOC Window File:
/***************************************************************************************************************
** Meta object code from reading C++ file 'window.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
***************************************************************************************************************/
#include "window.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'window.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_window_t {
QByteArrayData data[1];
char stringdata0[7];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_window_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_window_t qt_meta_stringdata_window = {
{
QT_MOC_LITERAL(0, 0, 6) // "window"
},
"window"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_window[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
0, 0, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
0 // eod
};
void window::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
Q_UNUSED(_o);
Q_UNUSED(_id);
Q_UNUSED(_c);
Q_UNUSED(_a);
}
QT_INIT_METAOBJECT const QMetaObject window::staticMetaObject = { {
&QWidget::staticMetaObject,
qt_meta_stringdata_window.data,
qt_meta_data_window,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *window::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *window::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_window.stringdata0))
return static_cast<void*>(this);
return QWidget::qt_metacast(_clname);
}
int window::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QWidget::qt_metacall(_c, _id, _a);
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Window Header File:
#ifndef WINDOW_H
#define WINDOW_H
#include
class window : public QWidget
{
Q_OBJECT
public:
explicit window(QWidget *parent = 0);
signals:
public slots:
protected:
void paintEvent(QPaintEvent *evnt);
};
#endif // WINDOW_H
Cubic Curve Pro File:
SOURCES += \
main.cpp \
window.cpp
QT = widgets
HEADERS += \
window.h
QT programming Example output:

Chapter 6 : Application Creation
Brief:
/*-----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of designing a mainwindow
* Description : This sample code shows how to build basic appication with minimum features
*---------------------------------------------------------------------------------------------------------------*/
#include <QtWidgets/QApplication>
#include "mainwindow.h"
int main(int argc, char** argv)
{
QApplication app(argc, argv);
MainWindow* win = new MainWindow;
win->resize(640, 480);
win->show();
return app.exec();
}
Main Window File:
/*-------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : window.cpp
* Title : A demo code of designing a mainwindow
* Description : This sample code shows how to build Menu bar and adding required shortcuts like "new file",
* "save" etc
*-----------------------------------------------------------------------------------------------*/
#include <QtWidgets/QLabel>
#include <QtWidgets/QAction>
#include <QtWidgets/QMenuBar>
#include <QtWidgets/QToolBar>
#include <QtWidgets/QStatusBar>
#include <QtWidgets/QDockWidget>
#include <QtWidgets/QListWidget>
#include <QtWidgets/QApplication>
#include <QtCore/QDebug>
#include "mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
setWindowTitle("Main Window Example");
// set the central widget
QLabel *label = new QLabel("Central Widget", this);
label->setAlignment(Qt::AlignCenter);
setCentralWidget(label);
// call varius setup functions
setupActions();
setupMenuBar();
setupToolBar();
setupStatusBar();
setupDockWindow();
}
void MainWindow::setupActions()
{
// create all actions and connect them with prepared slots
// the icons come from the oxygen theme and uses qt resource aliases
// without alias QIcon(":/icons/document-new.png")
m_fileNew = new QAction(QIcon(":new"), "New", this);
m_fileNew->setShortcuts(QKeySequence::New);
connect(m_fileNew, SIGNAL(triggered()), this, SLOT(onFileNew()));
m_fileOpen = new QAction(QIcon(":open"), "Open", this);
m_fileOpen->setShortcuts(QKeySequence::Open);
connect(m_fileOpen, SIGNAL(triggered()), this, SLOT(onFileOpen()));
m_fileSave = new QAction(QIcon(":save"), "Save", this);
m_fileSave->setShortcuts(QKeySequence::Open);
connect(m_fileSave, SIGNAL(triggered()), this, SLOT(onFileSave()));
m_fileSaveAs = new QAction(QIcon(":save-as"), "Save As", this);
m_fileSaveAs->setShortcuts(QKeySequence::SaveAs);
connect(m_fileSaveAs, SIGNAL(triggered()), this, SLOT(onFileSaveAs()));
m_fileExit = new QAction(QIcon(":exit"), "Exit", this);
m_fileExit->setShortcuts(QKeySequence::Quit);
connect(m_fileExit, SIGNAL(triggered()), qApp, SLOT(quit()));
m_editUndo = new QAction(QIcon(":undo"), "Undo", this);
m_editUndo->setShortcuts(QKeySequence::Undo);
m_editRedo = new QAction(QIcon(":redo"), "Redo", this);
m_editRedo->setShortcuts(QKeySequence::Redo);
m_editCut = new QAction(QIcon(":cut"), "Cut", this);
m_editCut->setShortcuts(QKeySequence::Cut);
m_editCopy = new QAction(QIcon(":copy"), "Copy", this);
m_editCopy->setShortcuts(QKeySequence::Copy);
m_editPaste = new QAction(QIcon(":paste"), "Paste", this);
m_editPaste->setShortcuts(QKeySequence::Cut);
m_helpAbout = new QAction("About", this);
connect(m_helpAbout, SIGNAL(triggered()), this, SLOT(onHelpAbout()));
m_helpAboutQt = new QAction("About Qt", this);
}
void MainWindow::setupMenuBar()
{
// add actions to the file, edit and help menus
m_fileMenu = menuBar()->addMenu("File");
m_fileMenu->addAction(m_fileNew);
m_fileMenu->addSeparator();
m_fileMenu->addAction(m_fileOpen);
m_fileMenu->addAction(m_fileSave);
m_fileMenu->addAction(m_fileSaveAs);
m_fileMenu->addSeparator();
m_fileMenu->addAction(m_fileExit);
m_editMenu = menuBar()->addMenu("Edit");
m_editMenu->addAction(m_editUndo);
m_editMenu->addAction(m_editRedo);
m_editMenu->addSeparator();
m_editMenu->addAction(m_editCut);
m_editMenu->addAction(m_editCopy);
m_editMenu->addAction(m_editPaste);
m_helpMenu = menuBar()->addMenu("Help");
m_helpMenu->addAction(m_helpAbout);
m_helpMenu->addAction(m_helpAboutQt);
}
void MainWindow::setupToolBar()
{
// create our only toolbar
m_toolBar = addToolBar("Standard");
m_toolBar->addAction(m_fileNew);
m_toolBar->addAction(m_fileOpen);
m_toolBar->addAction(m_fileSave);
}
void MainWindow::setupStatusBar()
{
// create statusbar and set message
QStatusBar *bar = statusBar();
// message will vanish after 10secs
bar->showMessage("Ready...", 10000);
}
void MainWindow::setupDockWindow()
{
// setup a dock window on the right with a list widget
QDockWidget *dock = new QDockWidget("DockWidget", this);
QListWidget *widget = new QListWidget(dock);
widget->addItem("Dock Content");
dock->setWidget(widget);
addDockWidget(Qt::LeftDockWidgetArea, dock);
}
void MainWindow::onFileNew()
{
qDebug() << "onFileNew()";
}
void MainWindow::onFileOpen()
{
qDebug() << "onFileOpen()";
}
void MainWindow::onFileSave()
{
qDebug() << "onFileSave()";
}
void MainWindow::onFileSaveAs()
{
qDebug() << "onFileSaveAs()";
}
void MainWindow::onHelpAbout()
{
qDebug() << "onHelpAbout()";
}
Main Window Header File:
/******************************************************************************************************************
*
* Copyright (c) 2012, Digia Plc.
* All rights reserved.
*
* See the LICENSE.txt file shipped along with this file for the license.
*
*****************************************************************************************************************/
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QtWidgets/QMainWindow>
class MainWindow : public QMainWindow
{
Q_OBJECT
public: // constructor
MainWindow(QWidget *parent = 0);
public: // setup functions
void setupActions();
void setupMenuBar();
void setupToolBar();
void setupStatusBar();
void setupDockWindow();
private slots:
void onFileNew();
void onFileOpen();
void onFileSave();
void onFileSaveAs();
void onHelpAbout();
private: // data member
QMenu *m_fileMenu;
QMenu *m_editMenu;
QMenu *m_helpMenu;
QToolBar *m_toolBar;
QAction *m_fileNew;
QAction *m_fileOpen;
QAction *m_fileSave;
QAction *m_fileSaveAs;
QAction *m_fileExit;
QAction *m_editUndo;
QAction *m_editRedo;
QAction *m_editCut;
QAction *m_editCopy;
QAction *m_editPaste;
QAction *m_helpAbout;
QAction *m_helpAboutQt;
};
#endif // MAINWINDOW_H
Main Window Pro File:
QT += widgets
SOURCES += \
main.cpp \
mainwindow.cpp
HEADERS += \
mainwindow.h
CONFIG -= app_bundle
RESOURCES += \
mainwindow.qrc
QT programming Example output:



Brief:
/*-----------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : main.cpp
* Title : A demo code of designing a window using QAction
* Description : This sample code contains 1 Menu bar with option.
*---------------------------------------------------------------------------------------------------------------*/
#include "mainwindow.h"
#include
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
Main Window File:
/*------------------------------------------------------------------------------------------------------------------
* Author : Emertxe (https://emertxe.com)
* Date : Wed 08 May 2021 16:00:04 IST
* File : mainwindow.cpp
* Title : A demo code of designing a window using QAction
* Description : This sample code contains 1 Menu bar with option. Based on used input,
* "Triggered" message will be displaed. A window is designed using Forms
*----------------------------------------------------------------------------------------------------------------*/
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
QMenu *fileMenu = new QMenu(this);
fileMenu->setTitle("File");
QAction *newAction = new QAction(this);
newAction->setText("New");
fileMenu->addAction(newAction);
ui->menuBar->addMenu(fileMenu);
QObject::connect(newAction, SIGNAL(triggered(bool)), this, SLOT(on_newAction_triggered(bool)));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_newAction_triggered(bool)
{
ui->label->setText("Triggered");
}
MOC Main Window File:
/**************************************************************************************************************
** Meta object code from reading C++ file 'mainwindow.h'
**
** Created by: The Qt Meta Object Compiler version 67 (Qt 5.12.8)
**
** WARNING! All changes made in this file will be lost!
**************************************************************************************************************/
#include "mainwindow.h"
#include <QtCore/qbytearray.h>
#include <QtCore/qmetatype.h>
#if !defined(Q_MOC_OUTPUT_REVISION)
#error "The header file 'mainwindow.h' doesn't include ."
#elif Q_MOC_OUTPUT_REVISION != 67
#error "This file was generated using the moc from 5.12.8. It"
#error "cannot be used with the include files from this version of Qt."
#error "(The moc has changed too much.)"
#endif
QT_BEGIN_MOC_NAMESPACE
QT_WARNING_PUSH
QT_WARNING_DISABLE_DEPRECATED
struct qt_meta_stringdata_MainWindow_t {
QByteArrayData data[3];
char stringdata0[35];
};
#define QT_MOC_LITERAL(idx, ofs, len) \
Q_STATIC_BYTE_ARRAY_DATA_HEADER_INITIALIZER_WITH_OFFSET(len, \
qptrdiff(offsetof(qt_meta_stringdata_MainWindow_t, stringdata0) + ofs \
- idx * sizeof(QByteArrayData)) \
)
static const qt_meta_stringdata_MainWindow_t qt_meta_stringdata_MainWindow = {
{
QT_MOC_LITERAL(0, 0, 10), // "MainWindow"
QT_MOC_LITERAL(1, 11, 22), // "on_newAction_triggered"
QT_MOC_LITERAL(2, 34, 0) // ""
},
"MainWindow\0on_newAction_triggered\0"
};
#undef QT_MOC_LITERAL
static const uint qt_meta_data_MainWindow[] = {
// content:
8, // revision
0, // classname
0, 0, // classinfo
1, 14, // methods
0, 0, // properties
0, 0, // enums/sets
0, 0, // constructors
0, // flags
0, // signalCount
// slots: name, argc, parameters, tag, flags
1, 1, 19, 2, 0x0a /* Public */,
// slots: parameters
QMetaType::Void, QMetaType::Bool, 2,
0 // eod
};
void MainWindow::qt_static_metacall(QObject *_o, QMetaObject::Call _c, int _id, void **_a)
{
if (_c == QMetaObject::InvokeMetaMethod) {
auto *_t = static_cast(_o);
Q_UNUSED(_t)
switch (_id) {
case 0: _t->on_newAction_triggered((*reinterpret_cast< bool(*)>(_a[1]))); break;
default: ;
}
}
}
QT_INIT_METAOBJECT const QMetaObject MainWindow::staticMetaObject = { {
&QMainWindow::staticMetaObject,
qt_meta_stringdata_MainWindow.data,
qt_meta_data_MainWindow,
qt_static_metacall,
nullptr,
nullptr
} };
const QMetaObject *MainWindow::metaObject() const
{
return QObject::d_ptr->metaObject ? QObject::d_ptr->dynamicMetaObject() : &staticMetaObject;
}
void *MainWindow::qt_metacast(const char *_clname)
{
if (!_clname) return nullptr;
if (!strcmp(_clname, qt_meta_stringdata_MainWindow.stringdata0))
return static_cast<void*>(this);
return QMainWindow::qt_metacast(_clname);
}
int MainWindow::qt_metacall(QMetaObject::Call _c, int _id, void **_a)
{
_id = QMainWindow::qt_metacall(_c, _id, _a);
if (_id < 0)
return _id;
if (_c == QMetaObject::InvokeMetaMethod) {
if (_id < 1)
qt_static_metacall(this, _c, _id, _a);
_id -= 1;
} else if (_c == QMetaObject::RegisterMethodArgumentMetaType) {
if (_id < 1)
*reinterpret_cast<int*>(_a[0]) = -1;
_id -= 1;
}
return _id;
}
QT_WARNING_POP
QT_END_MOC_NAMESPACE
Main Window Header File:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private:
Ui::MainWindow *ui;
public slots:
void on_newAction_triggered(bool);
};
#endif // MAINWINDOW_H
UI Main Window Header File:
/****************************************************************************************************************
** Form generated from reading UI file 'mainwindow.ui'
**
** Created by: Qt User Interface Compiler version 5.12.8
**
** WARNING! All changes made in this file will be lost when recompiling UI file!
****************************************************************************************************************/
#ifndef UI_MAINWINDOW_H
#define UI_MAINWINDOW_H
#include <QtCore/QVariant>
#include <QtWidgets/QAction>
#include <QtWidgets/QApplication>
#include <QtWidgets/QLabel>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QMenuBar>
#include <QtWidgets/QStatusBar>
#include <QtWidgets/QToolBar>
#include <QtWidgets/QWidget>
QT_BEGIN_NAMESPACE
class Ui_MainWindow
{
public:
QAction *actionNew;
QAction *actionLoad;
QAction *actionSave;
QAction *actionExit;
QAction *actionUndo;
QAction *actionRedo;
QAction *actionUndo_2;
QWidget *centralWidget;
QLabel *label;
QToolBar *mainToolBar;
QStatusBar *statusBar;
QMenuBar *menuBar;
void setupUi(QMainWindow *MainWindow)
{
if (MainWindow->objectName().isEmpty())
MainWindow->setObjectName(QString::fromUtf8("MainWindow"));
MainWindow->resize(400, 300);
actionNew = new QAction(MainWindow);
actionNew->setObjectName(QString::fromUtf8("actionNew"));
actionLoad = new QAction(MainWindow);
actionLoad->setObjectName(QString::fromUtf8("actionLoad"));
actionSave = new QAction(MainWindow);
actionSave->setObjectName(QString::fromUtf8("actionSave"));
actionExit = new QAction(MainWindow);
actionExit->setObjectName(QString::fromUtf8("actionExit"));
actionUndo = new QAction(MainWindow);
actionUndo->setObjectName(QString::fromUtf8("actionUndo"));
actionRedo = new QAction(MainWindow);
actionRedo->setObjectName(QString::fromUtf8("actionRedo"));
actionUndo_2 = new QAction(MainWindow);
actionUndo_2->setObjectName(QString::fromUtf8("actionUndo_2"));
centralWidget = new QWidget(MainWindow);
centralWidget->setObjectName(QString::fromUtf8("centralWidget"));
label = new QLabel(centralWidget);
label->setObjectName(QString::fromUtf8("label"));
label->setGeometry(QRect(100, 100, 47, 13));
MainWindow->setCentralWidget(centralWidget);
mainToolBar = new QToolBar(MainWindow);
mainToolBar->setObjectName(QString::fromUtf8("mainToolBar"));
MainWindow->addToolBar(Qt::TopToolBarArea, mainToolBar);
statusBar = new QStatusBar(MainWindow);
statusBar->setObjectName(QString::fromUtf8("statusBar"));
MainWindow->setStatusBar(statusBar);
menuBar = new QMenuBar(MainWindow);
menuBar->setObjectName(QString::fromUtf8("menuBar"));
menuBar->setGeometry(QRect(0, 0, 400, 21));
MainWindow->setMenuBar(menuBar);
retranslateUi(MainWindow);
QMetaObject::connectSlotsByName(MainWindow);
} // setupUi
void retranslateUi(QMainWindow *MainWindow)
{
MainWindow->setWindowTitle(QApplication::translate("MainWindow", "MainWindow", nullptr));
actionNew->setText(QApplication::translate("MainWindow", "New", nullptr));
actionLoad->setText(QApplication::translate("MainWindow", "Load", nullptr));
actionSave->setText(QApplication::translate("MainWindow", "Save", nullptr));
actionExit->setText(QApplication::translate("MainWindow", "Exit", nullptr));
actionUndo->setText(QApplication::translate("MainWindow", "Undo", nullptr));
actionRedo->setText(QApplication::translate("MainWindow", "Redo", nullptr));
actionUndo_2->setText(QApplication::translate("MainWindow", "Undo", nullptr));
label->setText(QApplication::translate("MainWindow", "Action", nullptr));
} // retranslateUi
};
namespace Ui {
class MainWindow: public Ui_MainWindow {};
} // namespace Ui
QT_END_NAMESPACE
#endif // UI_MAINWINDOW_H
Quaction Pro File:
#-------------------------------------------------
#
# Project created by QtCreator 2017-07-15T15:21:53
#
#-------------------------------------------------
QT += core gui
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
TARGET = Qaction
TEMPLATE = app
SOURCES += main.cpp\
mainwindow.cpp
HEADERS += mainwindow.h
FORMS += mainwindow.ui
QT programming Example output:
