Linux Internals Programming Assignments and Exercises
Linux Internals and TCP/IP Networking – Assignments
Linux Internals Programming Assignments will ensure you apply the concepts you have learned in your classroom. By solving these assignments, you will go through a systematic problem-solving approach, which includes requirement understanding, algorithm design, pseudocode creation, dry run, and final execution. As you move from simple to more complex assignments of each module, it will slowly start building your self-confidence. Explore the additional dimension with hands-on learning through Linux Internals Exercises to further enhance your mastery.
Chapter 1 : System Calls usage in Linux
Prerequisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Command line arguments, File operation system calls (open, read, write, close, fstat ..etc)
Objective:
- To understand and implement using basic system calls.
Requirements:
- Copy source file to destination file which passed through cmd-line arguments. After copying both files must have equal size, even it’s a zero sized file.
Eg: – ./my_copy source.txt dest.txt. - If arguments are missing show the usage (help) info to user.
- Implement a my_copy() function where you have to pass two file descriptors.
Int my_copy(int source_fd, int dest_fd); - If –p option passed copy permissions as well to destination (refer ‘fstat’ man page).
Eg: – ./my_copy –p source.txt dest.txt. - If the destination file is not present then create a new one with given name. Incase if it is present show a confirmation from user to overwrite.
Eg: – ./my_copy source.txt destination.txt
File “destination.txt” is already exists.
Do you want to overwrite (Y/n) - If user type ‘Y/n’ or enter key overwrite the destination with new file. In n/N don’t overwrite and exit from program.
- Program should able handle all possible error conditions.
Sample Execution / Output:
Test case 1

Test case 2

Test case 3

Test case 4

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Command line arguments, File operation system calls (open, read, write, close ..etc)
- Working of wc command
Objective:
- To understand and implement using basic system calls.
Requirements:
- Count the number of words, lines and characters(bytes) from files passed through command line.
- If more than one files passed, print individual count values and file name + calculate the total of all values and print at the end.
- If no file are passed wc will read from std input till end of file(Ctrl + D) then count lines, words and characters(bytes).
- Implement a word_count() function where you have to pass fd and 3 integer addresses(pass by refference). Int word_count (int fd, int *lines, int *words, int *bytes);
- Word_count function will read from the fd and calculates lines, words and bytes, then stores into respective addresses passed (don’t print values inside function).
- Main function will open the files in a loop and call word_count function depends upon number of files passed. Print values after calling functions in main.
Eg: – ./word_count file1.txt file2.txt
for(I = 0; I < 2; i++)
{
.
.
fd = open(…);
word_count(fd, &lines, &words, &bytes);
print_values(…);
.
.
}
Print_total(…); - If options passed [ -l –w –c ] print only respective values.
- Program should able to handle all possible error conditions.
Sample Execution / Output:
Test case 1

Test case 2

Test case 3
Test case 4
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Command line arguments, File operation system calls (open, read, write, close ..etc)
- Working of dup system calls.
Objective:
- To understand and implement using basic system calls.
Requirements:
- Using dup or dup2 redirect printf out to a given file instead of printing to stdout.
- Pass the file name using command-line arguments.
- Try using both system calls (dup and dup2).
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Command line arguments, File operation system calls (open, read, write, close ..etc)
- Working of fcntl system calls.
Objective:
- To understand and implement using advanced system calls.
- Understand the need of file synchronization between processes.
Requirements:
- Using fcntl system call synchronize a file between two processes (parent and child process).
- Pass the file name using command-line arguments.
- Before writing to file check file is locked, in case it is locked must wait the process until its unlocked.
- If its unlocked, lock file and continue with writing.
- Both process will do the same procedure.
Chapter 2 : Threads
Pre-requisites:
- Knowledge about multi-thread process, How to read and understand ‘man pages’.
- Good knowledge about pthread library functions.
Objective:
- To understand working and flow of multithread programs.
Requirements:
- Modify the factorial template code using multiple threads.
- Create at-least 3 threads and share the work among threads equally.
- Create and join threads using separate loops.
- You may have to change the argument structure.
- Declare all integer variables as unsigned long int (For max values).
Sample Execution / Output:
Test Case 1:

Pre-requisites:
- Knowledge about multi-thread process, How to read and understand ‘man pages’.
- Good knowledge about pthread library functions.
Objective:
- To understand working and flow of multithread programs.
Requirements:
- Modify the factorial template code using multiple threads.
- Create at-least 3 threads and share the work among threads equally.
- Create and join threads using separate loops.
- You may have to change the argument structure.
- Declare all integer variables as unsigned long int (For max values).
Sample Execution / Output:
Test Case 1:

Pre-requisites:
- Knowledge about multi-thread process, How to read and understand ‘man pages’.
- Good knowledge about pthread library functions.
- Multiplication of two matrices.
- Dynamic allocation for 2D array.
Objective:
- To understand working and flow of multithread programs..
Requirements:
- Create three local matrices, M1 MxN M2 NxP and Result MxP (M1 columns = M2 rows) where M, N & P values are provided by user.
- In case M1 columns != M2 rows print error message to user.
- Create all matrices using dynamic allocation.
- Use structure to pass arguments to threads
sample structure.
typedef struct thread_data
{
short m1_row;
short m1_col; short m2_col;int **matrix1;int **matrix2;
int **result;
short cur_row;
}Thread_data_t;
- Each thread will calculate and store single row in result. So number of threads equals number of rows in M1.
Eg: - M1 =
1 2 3 1 1 1 2 2 2 M2 =
1 1 1 2 2 2 3 3 3 Thread1 → M1 row1 x M2 col1, col2, col3
1×1 + 2×2 + 3×3 1×1 + 2×2 + 3×3 1×1 + 2×2 + 3×3 14 14 14 Thread2 → M1 row2 x M2 col1, col2, col3
1×1 + 1×2 + 1×3 1×1 + 1×2 + 1×3 1×1 + 1×2 + 1×3 6 6 6 Thread3 → M1 row3 x M2 col1, col2, col3
2×1 + 2×2 + 2×3 2×1 + 2×2 + 2×3 2×1 + 2×2 + 2×3 12 12 12 - Don’t create any global variables.
- Create generic function for matrix dynamic allocation and deallocating.
Sample Execution / Output:
Test Case 1:
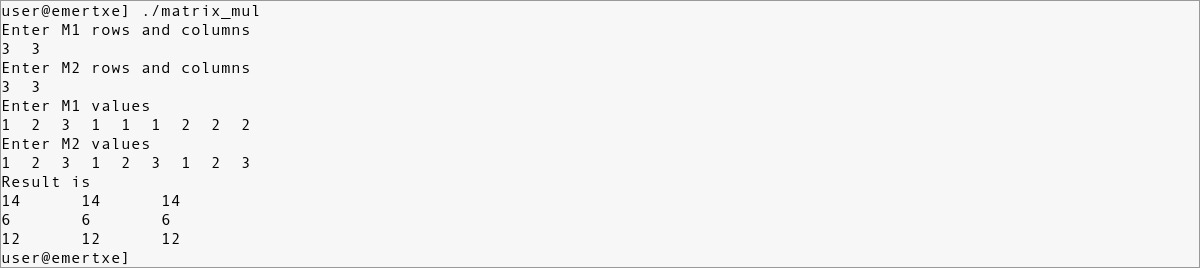
Chapter 3 : Signals
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about signals and signal handling.
- Working of sigaction system calls.
Objective:
- To understand working of signal handling.
Requirements:
- Write a signal handler function to print address which caused seg-fault(SIGSEGV).
- Use sigaction system call to register signal.
- Use struct siginfo from sa_sigaction to print address (Read man page).
- Create a segmentation fault from code.
- When error occurs program should print address and exit.
Sample Execution / Output:
Test case 1:

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about signals and signal handlers.
- Working of alarm system calls.
Objective:
- To understand signals and time related system calls.
Requirements:
- User gives the time and date from command-line arguments.
- Validate the time eg: Do not go behind the current time.
- Date is an option, if user not providing date consider it as today.
- In handler, avoid all user communication(printf, scanf etc) and loops. Make it minimal as possible.
- After the alarm expires, display a ALARM message along with date and time.
- Prompt the user whether he wants to stop or snooze.
- If user selects stop, terminate the program.
- If user selects snooze, prompt for snooze time in minutes.
> If user enters the time, reset the alarm to the entered time in minutes
> If user doesn’t enter time, default the snooze time to 1 mins
Sample Execution / Output:
Test case 1
Test case 2

Test case 3

Test case 4
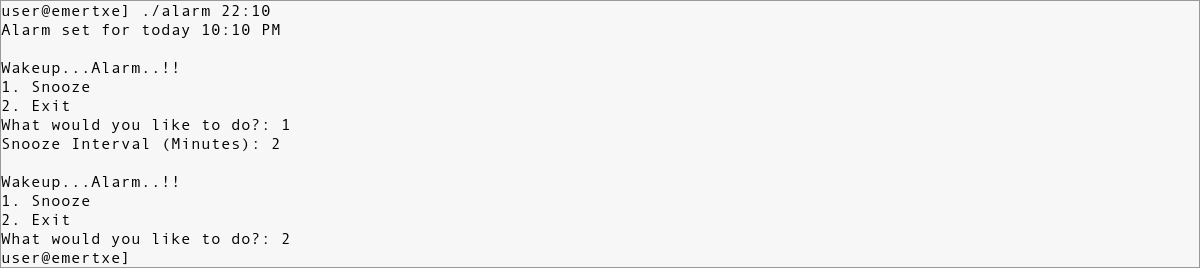
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about signals and signal handling.
- Working of sigaction system call and signal masking.
Objective:
- To understand importance of signal masking.
Requirements:
- Write a signal handler function for any signal, say SIGINT .
- While its running inside handler (use loop) block other signals(atleast 3) being received.
- Use sa_mask from struct sigaction to set signals to be blocked (Refer man ).
- To create a signal set use variable of sigset_t.
- To add or remove signals from set use sigaddset, sigdelset functions (refer man).
- Process will receive blocked signals once its out from handler.
Sample Execution / Output:
Test case 1
Test case 2

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about signals and signal handling.
- Working of sigaction system call.
Objective:
- To understand, how to avoid zombie asynchronously
Requirements:
Write two separate programs for both methods.
Method 1
- Create a child process.
- Write a signal handler function for SIGCHLD to avoid child become zombie (Do man 7 signal for SIGCHLD).
- Write code inside handler to avoid zombie and print child exit status.
Method 2
- Create a child process.
- Use sa_flag from struct sigaction to avoid zombie (Refer man ).
- Prints the child exit status inside handler.
Sample Execution / Output:
Test case 1

Chapter 4 : Process
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes, zombie and orphan.
- Working of fork system call.
Objective:
- To understand different states of a process.
Requirements:
- Create a child process and print status that process is running
- After some time print status that process is zombie state
- After some time print zombie process cleared by init.
- To print status use help of /proc//status file.
For eg: if child pid is 1234, open file /proc/1234/status and print first 3 lines - If that file is not available means that process is cleared.
Sample Execution / Output:
Test case 1

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes & zombie process.
- Working of fork & wait system call.
Objective:
- To understand different states of a process.
Requirements:
- Create a child process avoid it become a zombie.
- To avoid zombie we need to call wait(), but this block parent until child terminates.
- So we need to use waitpid() with proper arguments (Read man page).
- When child is working parent has to continuously print some message.
- When ever child terminates parent has to print child terminated and print exit status of child process.
Sample Execution / Output:
Test case 1

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes.
- Working of fork, wait and exec system calls.
Objective:
- To understand how to use exec system calls.
Requirements:
- Create child and execute a command passed from command-line arguments.
- If no arguments passed print a usage message.
- In case any wrong command passed, print an error message.
- After child terminates print the exit status of child process.
Sample Execution / Output:
Test case 1

Test case 2
Test case 3

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes.
- Working of fork & wait system calls.
Objective:
- To understand how to use fork system calls.
Requirements:
- Create three child process from same parent.
- Parent has to wait for all three child process.
- Print exit status of each child when they terminates.
Sample Execution / Output:
Test case 1

Chapter 5 : IPC
Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes and IPC.
- Working of pipe & dup system calls.
Objective:
- To understand working of pipe between two process.
Requirements:
- Create two child process and execute commands passed from command-line arguments
- Each command is separated by a ‘|’ (pipe) character.
- First child execute first command (with or without options) and pass o/p to next.
- Second child executes second command (after ‘|’) will reads I/p from first cmd.
- Parent will wait for both child process to finish.
Sample Execution / Output:
Test case 1

Test case 2

Test case 3

Test case 4

Pre-requisites:
- Knowledge about system calls, How to read and understand ‘man pages’.
- Good knowledge about processes and IPC.
- Working of pipe & dup system calls.
Objective:
- To understand working of multiple pipes between multiple process.
Requirements:
- Create three child process and execute commands passed from command-line arguments
- Each command is separated by a ‘|’ (pipe) character.
- First child execute first command (ls -l) and pass output to next command.
- Second child executes second command (grep pattern) where pattern passed from command-line arguments.
- Third child executes wc -l
Sample Execution / Output:
Test case 1

Test case 2

Test case 3
